iOS Programming 101: Integrate Twitter and Facebook Sharing in Your App
Following the Twitter support in iOS 5, Apple added the support of Facebook in iOS 6. In the past, developers have to make use of the Facebook and Twitter API to integrate the sharing feature in their apps. With the built-in support, it’s much easier to add these social features to your own app.
In this short tutorial, we will show you how to build Twitter and Facebook sharing feature in your app. As usual, we’ll build a simple app together as we believe there is no better way to teach programming than “get your hand dirty”.
Social Framework in iOS 6
The iOS 6 introduces a new framework known as the “Social Framework”. The Social framework lets you integrate your app with any supported social networking services. Presently, it supports Facebook, Twitter and Sina Weibo. The framework gives you a standard interface to interact with different social networks and it shields you from learning the internals. You don’t have to worry about the Twitter/Facebook APIs, how to handle network connection, handle single sign-on, etc. The social framework simplifies everything. You just need to write a few lines of code to bring up the composer and users can tweet / publish Facebook post within your app.
The framework comes with a very handy class named SLComposeViewController. The SLComposeViewController class presents a standard view controller for users to compose tweet or Facebook post. It also allows developers to preset the initial text, attach images and add URL to the post. If you just want to implement simple sharing feature, this is the only class you need to know and that we will go through today.
If you’re not familiar with the SLComposeViewController, this is what it looks like within your app.
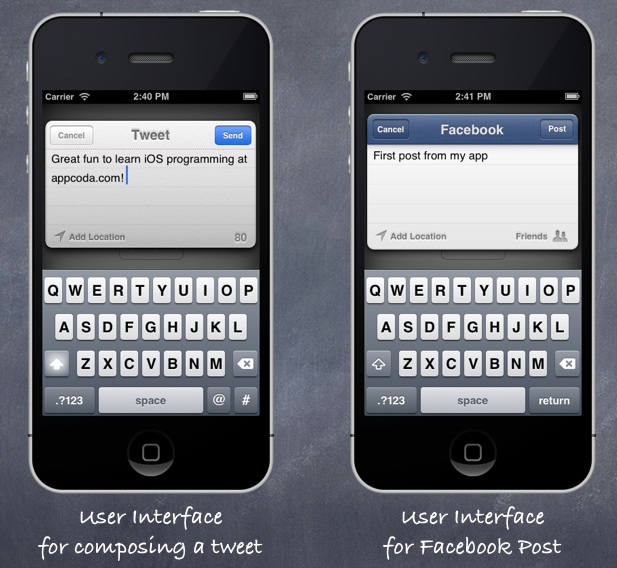
User Interface for Twitter and Facebook in Your App
Now you should have a basic idea about the framework. Let’s get started and build a simple app with Twitter and Facebook integration.
Create the Project and Design the Interface
First, create a new Xcode project using the Single View Template. Let’s name the project as “SocialSharing” and set the project with the following parameters:

SocialSharing Xcode Project
Once you’ve successfully created your project, go to the Storyboard and design the user interface. In the view, add two buttons. Name one as “Tweet” and the other one as “Facebook”.

Designing the interface for the App
Establish the Connection Between Variables and UI Elements
Next, we’ll connect the UI elements with our code. In the Storyboard, select the view controller and switch to the Assistant Editor.
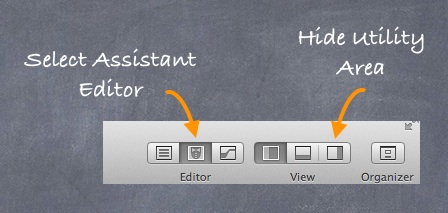
Show Assistant Editor and Hide Utility Area
Press and hold the control key, click the “Tweet” button and drag it towards the “SocialSharingViewController.h”. Create an action method and name it as “postToTwitter”. This method will be invoked when the button detects a Touch Up Inside event.

Add action methods for both Tweet and Facebook buttons
Repeat the same procedures the “Facebook” button and name the action method as “postToFacebook”. Below is the final code for “SocialSharingViewController.h”:
1 2 3 4 5 6 7 |
#import <UIKit/UIKit.h> @interface SocialSharingViewController : UIViewController - (IBAction)postToTwitter:(id)sender; - (IBAction)postToFacebook:(id)sender; @end |
Adding Twitter Support
Let’s first start with the implementation of “postToTwitter” method. Open the “SocialSharingViewController.m” and add the following code for the “postToTwitter” method:
1 2 3 4 5 6 7 8 9 |
- (IBAction)postToTwitter:(id)sender { if ([SLComposeViewController isAvailableForServiceType:SLServiceTypeTwitter]) { SLComposeViewController *tweetSheet = [SLComposeViewController composeViewControllerForServiceType:SLServiceTypeTwitter]; [tweetSheet setInitialText:@"Great fun to learn iOS programming at appcoda.com!"]; [self presentViewController:tweetSheet animated:YES completion:nil]; } } |
As “SLComposeViewController” is a class provided by the Social framework, remember to import the Social.h file at the very top of the “SocialSharingViewController.m”:
1 |
#import <Social/Social.h> |
Before we move on, let’s take a look at the above code line by line.
Line #2 – Firstly, we use the “isAvailableForServiceType” method to verify if the Twitter service is accessible. One reason why users can’t access the Twitter service is that they haven’t logged on the service in the iOS.
Line #4 – If the service is accessible, we then create an instance of the SLComposeViewController for the Twitter service.
Line #5 – We set the initial text in the composer.
Line #6 – Finally, we invoke presentViewController:tweetSheet to bring up the Twitter composer.
That’s the code we need to let user tweet within your own app. It’s much easier than you thought, right? But we still miss one thing before the app can be run.
By default, the Social framework is not included in the Xcode project. To compile the app properly, add the “Social.framework” in the project. In the Project Navigator, select the “SocialSharing” project. In the Content Area, select “SocialSharing” under Targets and click “Build Phases”. Expand “Link Binary with Libraries” and click the “+” button to add the “Social.framework”.

Add Social framework to the project
Now, it’s ready to test the app. Click the “Run” button to try out the app in Simulator. When the app is launched, tap the “Tweet” button and you should see a similar screenshot as below:

Testing the Tweet Feature
In case you haven’t registered your Twitter account in the Simulator, you’ll end up with the below. Just go to Settings -> Twitter and sign on with your Twitter account.
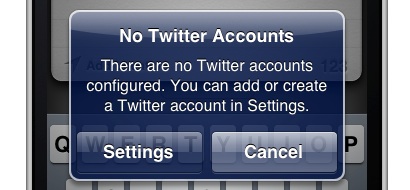
You haven’t registered your Twitter account in iOS
Adding Facebook Support
Next, we’ll implement the button for publishing wall post on Facebook. In the “SocialSharingViewController.m”, add the following code in the “postToFacebook” method:
1 2 3 4 5 6 7 8 |
- (IBAction)postToFacebook:(id)sender { if([SLComposeViewController isAvailableForServiceType:SLServiceTypeFacebook]) { SLComposeViewController *controller = [SLComposeViewController composeViewControllerForServiceType:SLServiceTypeFacebook]; [controller setInitialText:@"First post from my iPhone app"]; [self presentViewController:controller animated:YES completion:Nil]; } } |
That’s it. The code is very similar to the code we’ve used in the “postToTwitter” method. The only change is the service type. Instead of using SLServiceTypeTwitter, we tell SLComposeViewController to use SLServiceTypeFacebook.
Let’s run the app again and click the “Facebook” button. Your app should bring up the composer for publishing Facebook post.
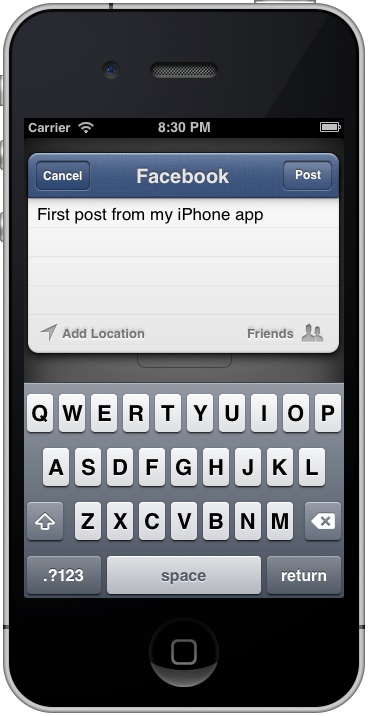
Adding Image and Link to Your Post
Wouldn’t be great to upload your post with image and link? The SLComposeViewController comes with some built-in methods to allow you easily do the upload. Let’s tweak the code of the Facebook button and make the upload work.
First, download this image and add it to the Xcode project. (You can use any image but make sure you name it as “socialsharing-facebook-image.jpg”)
You can simply use the “addImage” method to attach an image and the “addURL” method to include a link.
1 2 |
[controller addURL:[NSURL URLWithString:@"http://www.appcoda.com"]]; [controller addImage:[UIImage imageNamed:@"socialsharing-facebook-image.jpg"]]; |
The final code for “postToFacebook” should look like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
- (IBAction)postToFacebook:(id)sender { if([SLComposeViewController isAvailableForServiceType:SLServiceTypeFacebook]) { SLComposeViewController *controller = [SLComposeViewController composeViewControllerForServiceType:SLServiceTypeFacebook]; [controller setInitialText:@"First post from my iPhone app"]; [controller addURL:[NSURL URLWithString:@"http://www.appcoda.com"]]; [controller addImage:[UIImage imageNamed:@"socialsharing-facebook-image.jpg"]]; [self presentViewController:controller animated:YES completion:Nil]; } } |
Go ahead to run the app and test out the Facebook feature again. You should see a thumbnail and a link bundled in the post.

Upload Post with image and link
Summary
As you can see from this tutorial, it’s pretty easy to add Twitter and Facebook feature using the Social Framework in iOS 6. If you’re building your app, there is no reason why you shouldn’t incorporate the social features. With Twitter and Facebook support, it adds values to your app and may boost its popularity.
The tutorial introduces the basics of Facebook and Twitter integration. You can try to tweak the demo app and upload multiple images to the social networks. However, if you want to access more advanced features such as display users’ Facebook friends, you’ll need to study the Facebook API.
I hope you enjoy the tutorial and you have a better understanding about the social framework. Feel free to leave your questions and share your thought about the tutorial.