iOS Programming 101: Customize UITableView and UITableViewCell Background using Storyboard
In the last tutorial of our iOS Programming 101 series, we showed you how to customize the navigation bar and buttons with your own background image. This time, we’ll look into the customization and styling of UITableView and UITableViewCell.
If you’ve followed our iOS tutorials from the very beginning, you know we’ve written a tutorial about customizing table view cell using Interface Builder. In this tutorial, we’ll do something different. Instead of using Interface Builder to tweak the UITableViewCell, we’ll show you how to use Storyboards to style the cell. Storyboards make customizing table cells much easier with the introduction of prototype cell. In brief, you’ll learn the following stuffs in this tutorial:
- Customizing UITableViewCell using Storyboard
- Style UITableViewCell and UITableView with your own background images
To illustrate the customization, we’ll tweak a simple table view, style it and make it more attractive. To help you focus on learning the customization, we’ve prepared the Xcode project for you to start with. Before proceeding, first download the Xcode project here (note: the project is created using Xcode 4.5).
Designing Prototype Cells in Storyboard
Since the release of iOS 5, Apple introduced Storyboarding that simplifies the way to design user interfaces for your app. To customize a table cell, you no longer need to create a separate cell in Interface Builder. You can design the cell right inside the Storyboard editor.
Open the Xcode project you’ve downloaded. Go to the Storyboard and you should see something like this:

Table View Controller with Empty Prototype Cell
When you add a UITableViewController in the Story, by default, you should see an empty prototype cell. You can now add other UI control elements (e.g. UILabel, UIImageView) right into the prototype cell. Let’s first change the height of the cell. Select the Prototype Cells, click the “Size” inspector and change the row height from 44 to 71.
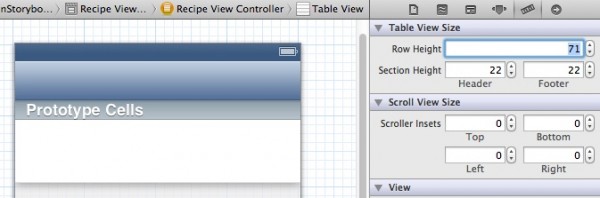
Changing row height in Storyboard
Next, drag an Image View from object library to the prototype cell. Again, select the image view, click “Size” inspector and change the “X”, “Y”, “Width” and “Height” attributes as follows:
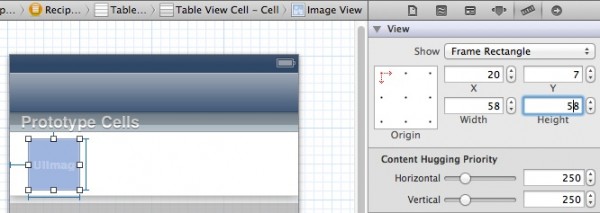
Add image view to prototype cell
Switch to the “Attributes” inspector, set the “Tag” to 100. You can think of a tag as an unique identifier of the UI control. Later in our code, we’ll use this tag to identify the image view.

Assigning a tag for the image view
Then drag a label to the cell and name it as “RecipeName”. This label is used to display the name of recipe. In the “Size” inspector, set “X” as 92, “Y” as 7, “Width” as 186 and “Height” as 21. In the “Attributes” inspector, change the font type to “Helvetica Neue Condensed Bold” and set the font size to 21 points. Finally, set the “Tag” value to 101.
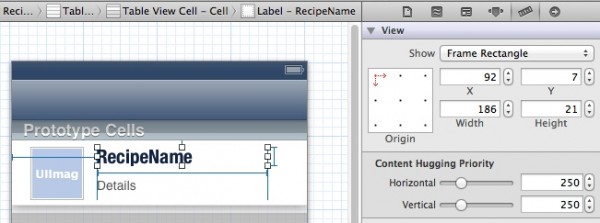
Add labels to the prototype cell
Add another label to the cell and name it as “Details”. In the “Size” inspector, set “X” as 92, “Y” as 32, “Width” as 186 and “Height” as 33. Switch to the “Attributes” inspector, set the “Tag” value as 102.
Coding the Table View Controller
With the user interface designed, let’s get back to the code. Open “RecipeViewController.m”, which is the corresponding class of the Table View Controller, change the “cellForRowAtIndexPath:” method to the following:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath { static NSString *CellIdentifier = @"Cell"; UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier]; // Configure the cell... if (cell == nil) { cell = [[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:CellIdentifier]; } // Display recipe in the table cell Recipe *recipe = [recipes objectAtIndex:indexPath.row]; UIImageView *recipeImageView = (UIImageView *)[cell viewWithTag:100]; recipeImageView.image = [UIImage imageNamed:recipe.imageFile]; UILabel *recipeNameLabel = (UILabel *)[cell viewWithTag:101]; recipeNameLabel.text = recipe.name; UILabel *recipeDetailLabel = (UILabel *)[cell viewWithTag:102]; recipeDetailLabel.text = recipe.detail; return cell; } |
We’ve added the code (from line 12 to 20) for displaying the cell labels and image view. As you can see from the code, we invoke the “viewWithTag:” method with the tag value to identify the UI component.
Compile and run the app. Your app should look like this:

Recipe Book App with default cell style
Styling the UITableCell with Background Image
Now you’ve learnt how to create custom table cell using Storyboard, but the table view cell is just in plain style. We want to change the look & feel of the table cells and make them prettier. In the Xcode project, you should find three background images for table cell. The images are specially designed for different types of cells.

Cell Background Image – Top and Bottom images are with rounded corners
The UITableViewCell class allows developers to assign a custom background view using the “backgroundView:” method. To assign your own custom background image, we can create an UIImageView object with the background image and set it as the background view of the cell. Add the following code to the “cellForRowAtIndexPath:” method:
1 2 3 4 5 6 |
// Assign our own background image for the cell UIImage *background = [self cellBackgroundForRowAtIndexPath:indexPath]; UIImageView *cellBackgroundView = [[UIImageView alloc] initWithImage:background]; cellBackgroundView.image = background; cell.backgroundView = cellBackgroundView; |
There is one thing missing. We haven’t implemented the “cellBackgroundForRowAtIndexPath:” method. As explained before, we have three types of background image. The top and bottom cells are assigned with different background images that are differed from the rest of the cells. So we create the “cellBackgroundForRowAtIndexPath:” method to determine the background image to assign. Add the following code before the “cellForRowAtIndexPath:” method:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
- (UIImage *)cellBackgroundForRowAtIndexPath:(NSIndexPath *)indexPath { NSInteger rowCount = [self tableView:[self tableView] numberOfRowsInSection:0]; NSInteger rowIndex = indexPath.row; UIImage *background = nil; if (rowIndex == 0) { background = [UIImage imageNamed:@"cell_top.png"]; } else if (rowIndex == rowCount - 1) { background = [UIImage imageNamed:@"cell_bottom.png"]; } else { background = [UIImage imageNamed:@"cell_middle.png"]; } return background; } |
For the first row of the cell (i.e. rowIndex == 0), the method returns the top cell image. If it’s the last table cell (i.e. rowIndex == rowCount – 1), the method returns the bottom cell image. For the rest of table cell, it simply returns the middle cell image.
Lastly, add the following line of code in the “viewDidLoad” method of RecipeViewController.m. This tells the table cell not to show the separator as our own cell images already come with the separator.
1 |
[self.tableView setSeparatorStyle:UITableViewCellSeparatorStyleNone]; |
Compile and run the app. You should end up with an app like this:

Recipe book app with custom background cell image
Styling the Table View Background
The app looks much better, right? Let’s make it even better by assigning a background image to the view. Add the following code to “viewDidLoad” method of RecipeViewController.m:
1 2 |
self.parentViewController.view.backgroundColor = [UIColor colorWithPatternImage:[UIImage imageNamed:@"common_bg"]]; self.tableView.backgroundColor = [UIColor clearColor]; |
As the RecipeViewController is a subclass of UITableViewController, we use “self.parentViewController” to access its parent view, followed by assigning the background. By default, the table view is displayed in white. We set its color to transparent so as not to block out the background of the parent view.
Before you run the app, we’d like to make one more change. The table view is now very close to the navigation bar. You may want to add padding at the top of the table view. To add padding, you can use the contentInset property to specify a buffer area around the content of the view. The contentInset property is a UIEdgeInsets struct with the fields top, bottom, left, right. Let’s add the following code in “viewDidLoad”:
1 2 |
UIEdgeInsets inset = UIEdgeInsetsMake(5, 0, 0, 0); self.tableView.contentInset = inset; |
The above code results in an additional buffer area that is 5 pixels at the top of the table view. Compile and run the app again. Your app should now be customized with padding and our own background image:

Recipe book app in better style
What’s Coming Next
Again, I hope you enjoy the tutorial and find it useful. For your complete reference, you can download the final Xcode project here.
Earlier, we already showed you how to customize the tab bar and navigation bar. Combining with this tutorial, you should be able to make your table-based app more attractive. Later, we’ll see how to style other UI elements. Stay tuned.
What do you think about this tutorial? As always, leave us comment and share your thought.