In iOS 16, SwiftUI comes with a new view called ShareLink
. When users tap on the share link, it presents a share sheet for users to share content to other applications or copy the data for later use.
The ShareLink
view is designed to share any types of data. In this tutorial, we will show you how to use ShareLink
to let users share text, URL, and images.
Basic Usage of ShareLink
Let’s begin with an example. To create a share link for sharing a URL, you can write the code like this:
struct ContentView: View {
private let url = URL(string: "https://www.appcoda.com")!
var body: some View {
VStack {
ShareLink(item: url)
}
}
}
SwiftUI automatically renders a Share button with a small icon.
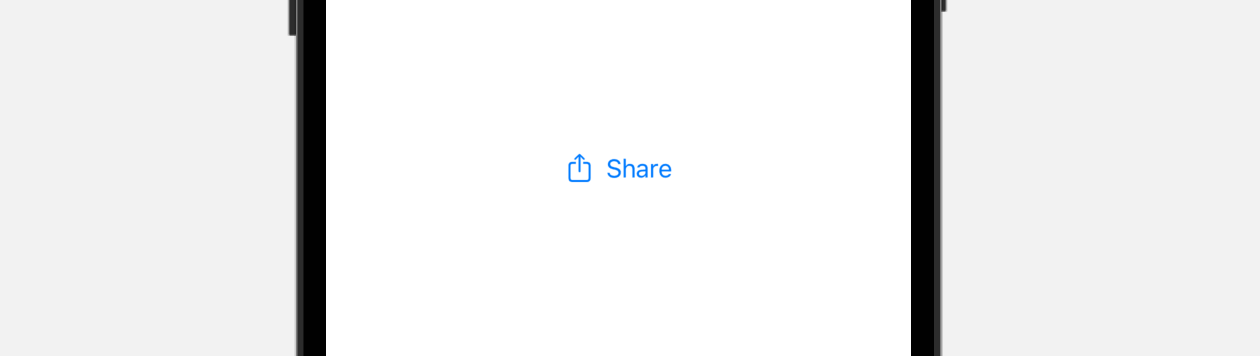
When tapped, iOS brings up a share sheet for users to perform further actions such as copy and adding the link to Reminders.
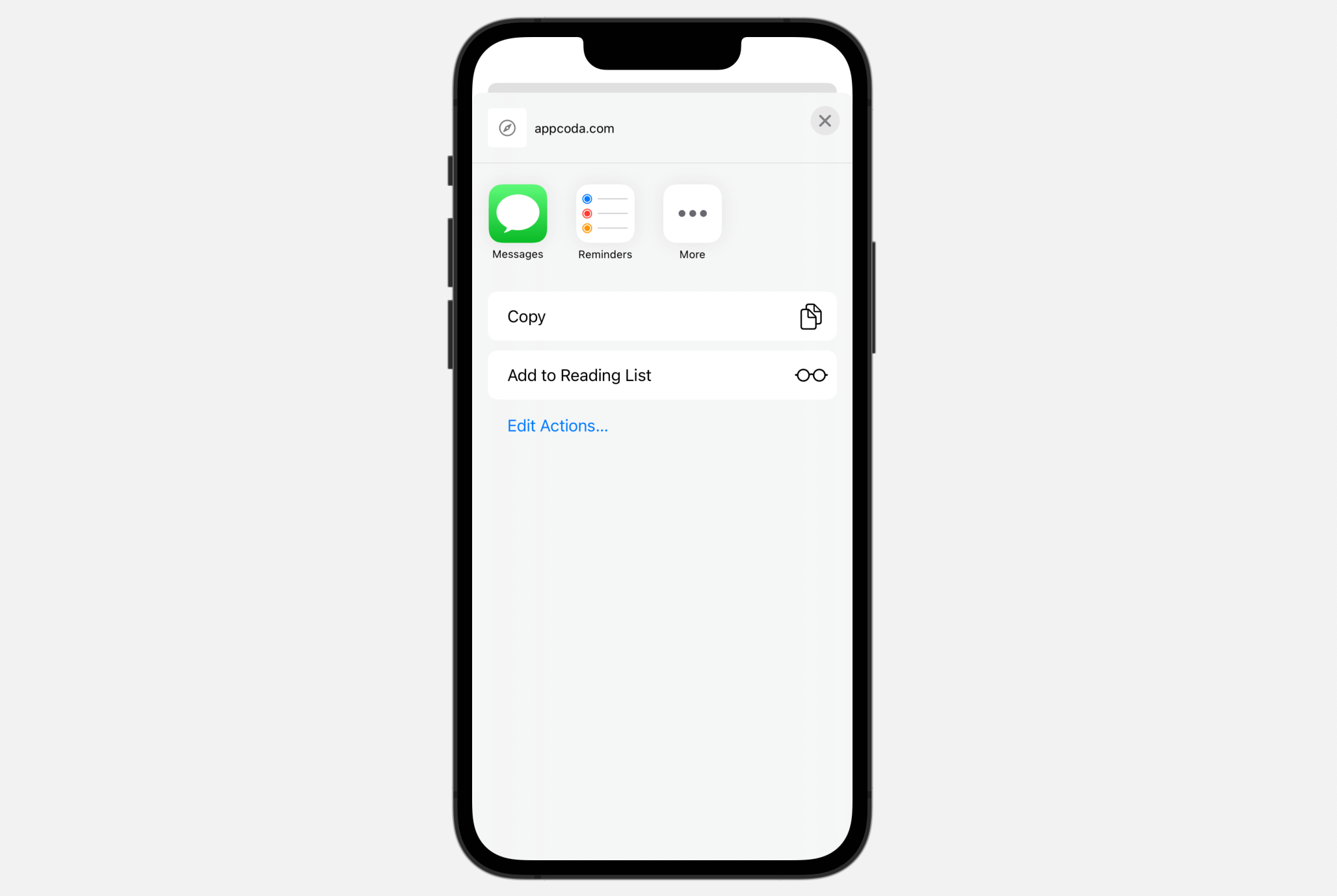
To share text, instead of URL, you can simply pass the a string to the item
parameter.
ShareLink(item: "Check out this new feature on iOS 16")
Customizing the Appearance of Share Link
To customize the appearance of the link, you can provide the view content in the closure like this:
ShareLink(item: url) {
Image(systemName: "square.and.arrow.up")
}
In this case, SwiftUI only displays the share icon for the link.
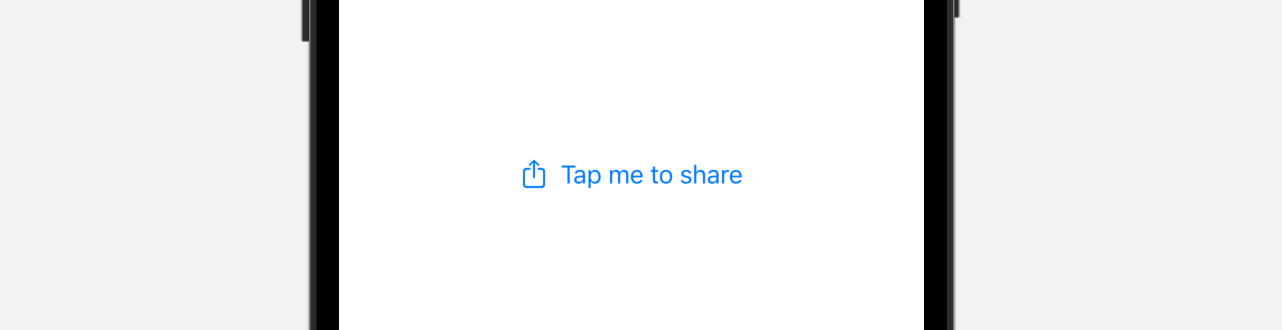
Alternatively, you can present a label with a system image or custom image:
ShareLink(item: url) {
Label("Tap me to share", systemImage: "square.and.arrow.up")
}
When initializing the ShareLink
instance, you can include two additional parameters to provide extra information about the shared item:
ShareLink(item: url, subject: Text("Check out this link"), message: Text("If you want to learn Swift, take a look at this website.")) {
Image(systemName: "square.and.arrow.up")
}
The subject
parameter lets you include a title about the URL or any item to share. The message
parameter allows you to specify the description of the item. Depending on the activities the user shares to, iOS will present the subject or message or both. Say, if you add the URL to Reminders, the Reminders app displays the preset message.
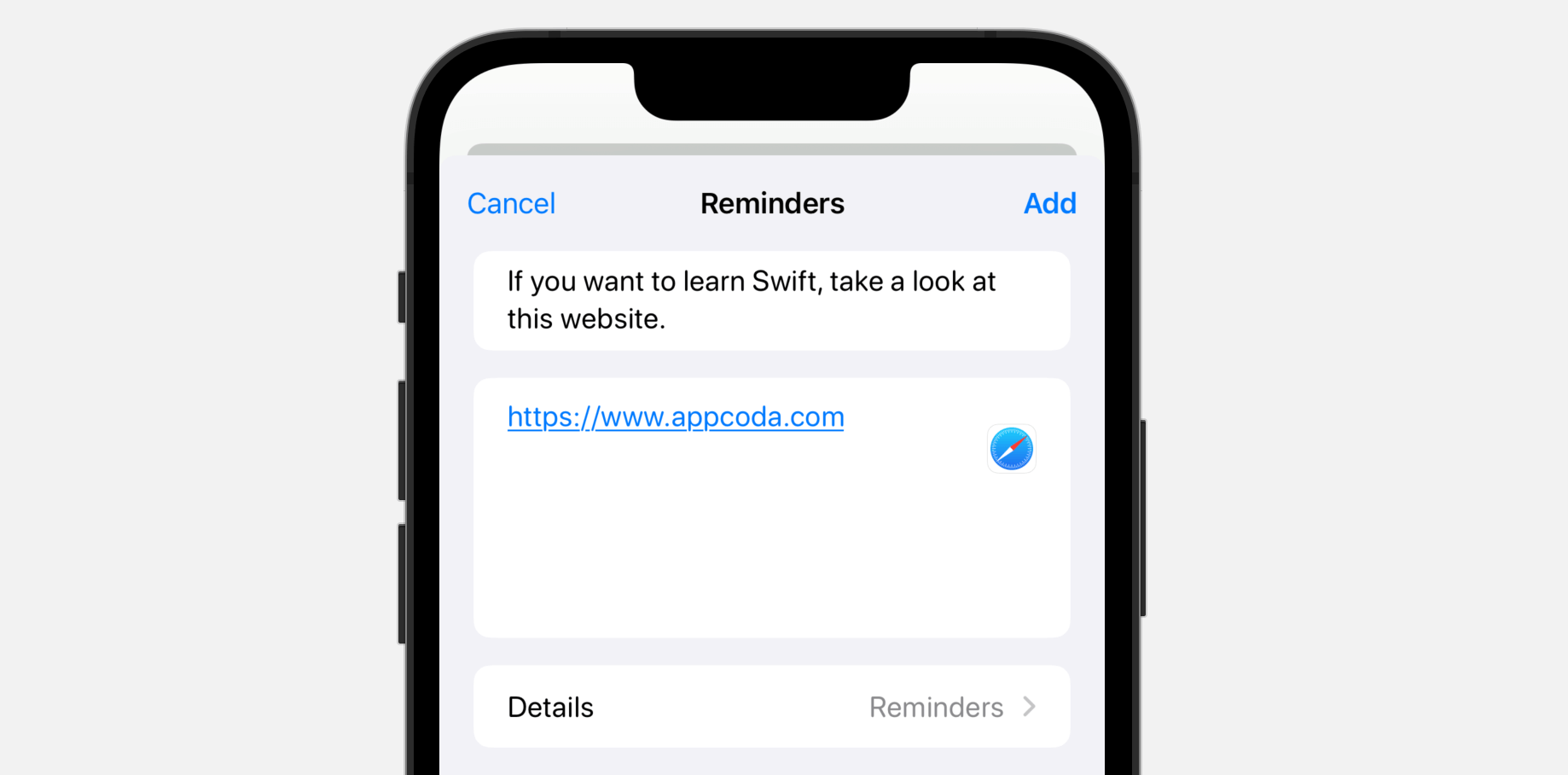
Sharing Images
Other than URLs, you can share images using ShareLink
. Here is a sample code snippet:
struct ContentView: View {
private let photo = Image("bigben")
var body: some View {
VStack(alignment: .leading, spacing: 10) {
photo
.resizable()
.scaledToFit()
ShareLink(item: photo, preview: SharePreview("Big Ben", image: photo))
}
.padding(.horizontal)
}
}
For the item
parameter, you specify the image to share. And, you provide a preview of the image by passing an instance of SharePreview
. In the preview, you specify the title of the image and the thumbnail. When you tap the Share button, iOS brings up a share sheet with the image preview.
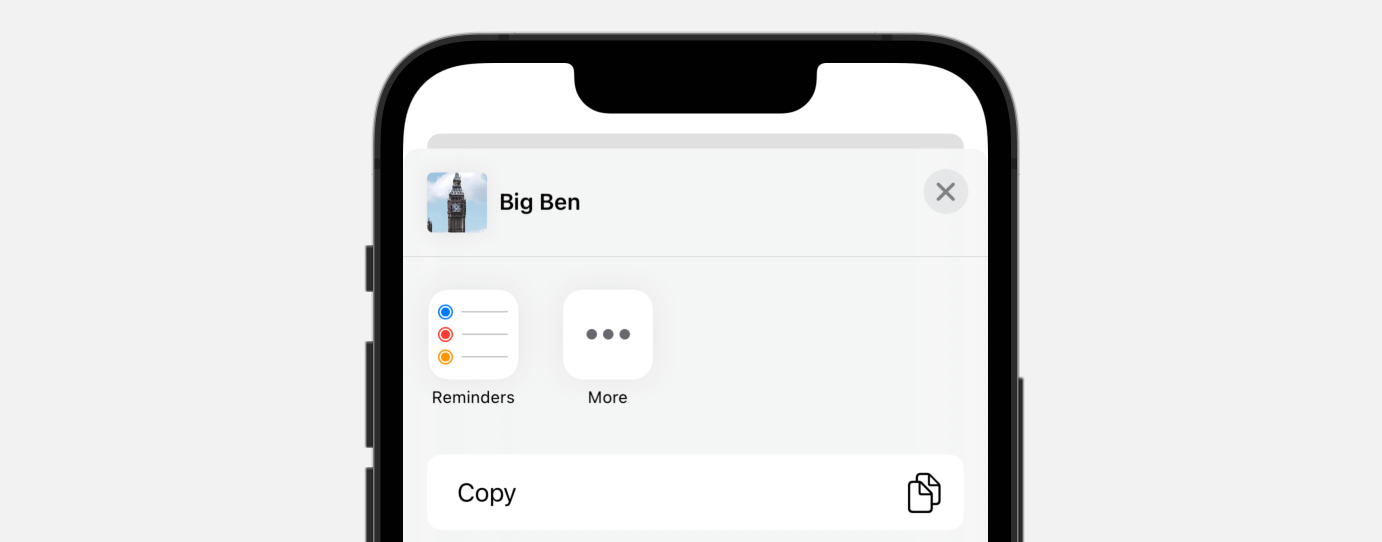
Conforming to Transferable
Other than URLs, the item
parameter accepts any objects that conforms to the Transferable
protocol. In iOS, the following types are the standard Transferable
types:
- String
- Data
- URL
- Attributed String
- Image
Transferable is a protocol that describes how a type interacts with transport APIs such as drag and drop or copy and paste.
So what if you have a custom object, how can you make it transferable? Let’s say, you create the following Photo
structure:
struct Photo: Identifiable {
var id = UUID()
var image: Image
var caption: String
var description: String
}
To let ShareLink
share this object, you have to adopt the Transferable
protocol for Photo
and implement the transferRepresentation
property:
extension Photo: Transferable {
static var transferRepresentation: some TransferRepresentation {
ProxyRepresentation(exporting: \.image)
}
}
There are a number of Transfer Representations including ProxyRepresentation
, CodableRepresentation
, DataRepresentation
, and FileRepresentation
. In the code above, we use the ProxyRepresentation
which is a transfer representation that uses another type’s transfer representation as its own. Here, we use the Image
‘s built-in Transferable
conformance.
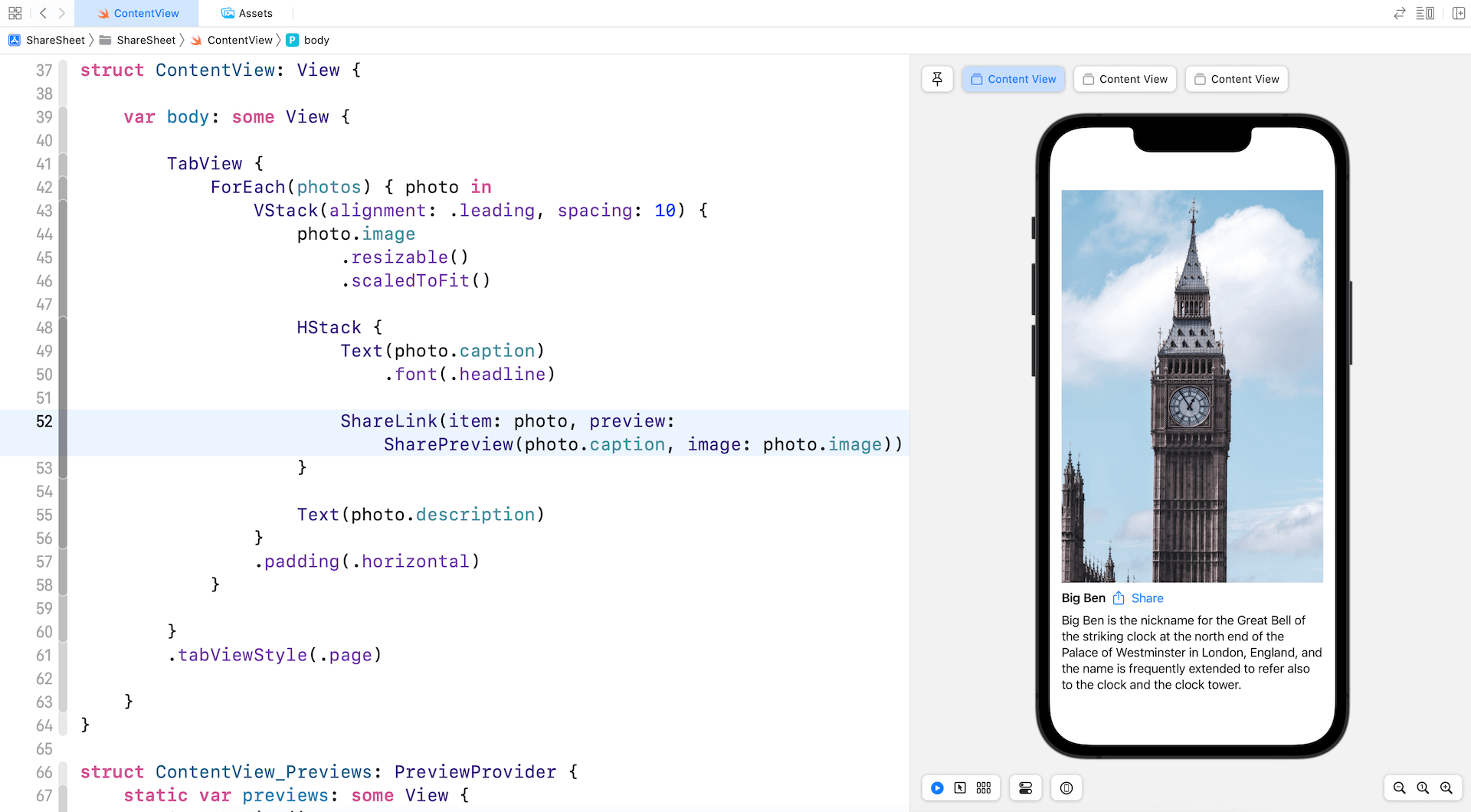
Since Photo
now conforms to Transferable
, you can pass the Photo
instance to ShareLink
:
ShareLink(item: photo, preview: SharePreview(photo.caption, image: photo.image))
When users tap the Share button, the app brings up the share sheet for sharing the photo.
What’s Next
This tutorial shows you how to use ShareLink
for sharing text, URL, and images. In fact, this new view allows you to share any types of data as long as the type conforms to the Transferable
protocol.
For custom types, you adopt the protocol and provide a transfer representation by using one of the built-in TransferRepresentation
types. We briefly discuss the ProxyRepresentation
type. If you need to share a file between applications, you can use the FileRepresentation
type. In later tutorials, we will discuss more on that.