How To Add a Slide-out Sidebar Menu in Your Apps
Editor’s note #1: This post has been updated for Xcode 6 and iOS 8. The demo app now supports the latest version of SWRevealViewController.
Editor’s note #2: If you’re using Swift, please check out the Swift version of the tutorial here.
How can I create a slide-out sidebar menu in my app? This is one of the most frequently asked questions we got from our readers. So this week we’ll show you how create a slide-out navigation menu similar to the one you find in the Facebook app.
For those who are unfamiliar with slide out navigation menu, Ken Yarmost gave a good explanation and defined it as follows:
Slide-out navigation consists of a panel that “slides out” from underneath the left or the right of the main content area, revealing a vertically independent scroll view that serves as the primary navigation for the application.
Since Facebook app introduced this slide-out sidebar design, it quickly becomes a standard way to implement navigation menu. You can easily find this design pattern in most of the popular content-related apps such as Path, Mailbox, Gmail, etc.
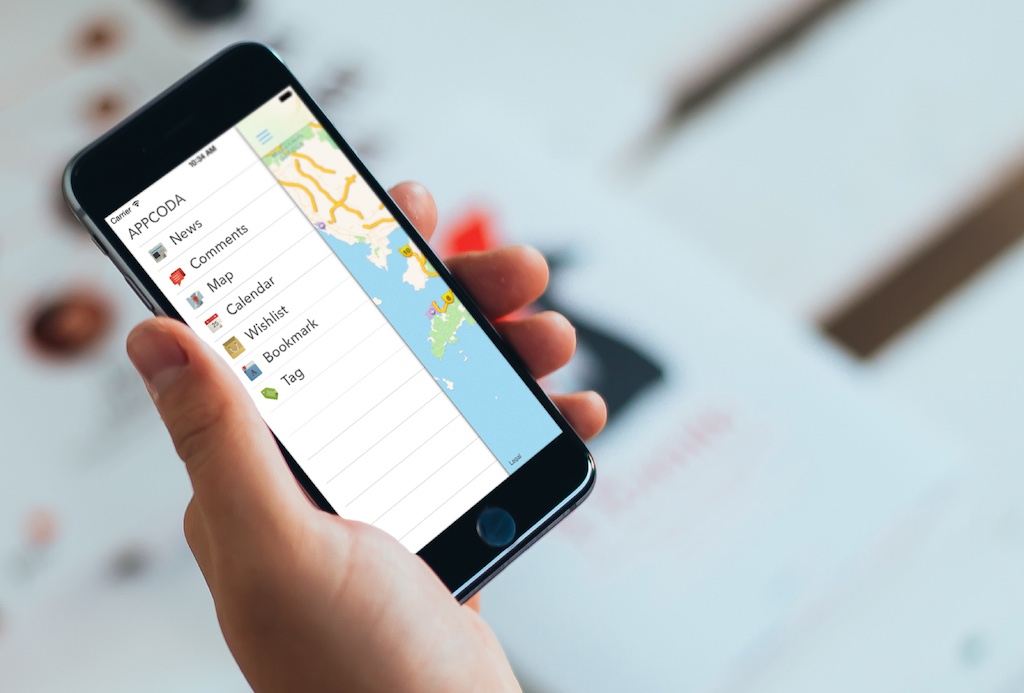
The slide-out design pattern lets you build a navigation menu in your apps but without wasting the screen real estate. Normally, the navigation menu is hidden behind the front view. The menu can then be triggered by tapping a list button in the navigation bar. Once the menu is expanded and becomes visible, users can close it by using the list button or simply swiping left on the content area.
With so many free pre-built solution on GitHub, we’re not going to build the slide-out navigation menu from scratch. Instead, we’ll make use of a library called SWRevealViewController. Developed by John Lluch, this excellent library provides a quick and easy way to put up a slide-out navigation menu and it’s available for free.
Read on and develop a demo app together.
A Glance at the Demo App
As usual, we’ll build a demo app to show you how to apply the SWRevealViewController. The app is very simple but not fully functional. The primary purpose of the app is to walk you through the implementation of slide-out navigation menu. The navigation menu will work like this:
- User triggers the menu by tapping the list button at the top-left of navigation bar.
- User can also bring up the menu by swiping right on the main content area.
- Once the menu appears, user can close it by tapping the list button again.
- User can also close the menu by dragging left on the content area.

Creating the Xcode Project
With a basic idea about what we’ll build, let’s move on. You can create the Xcode project from scratch and design the user interface similar to below:
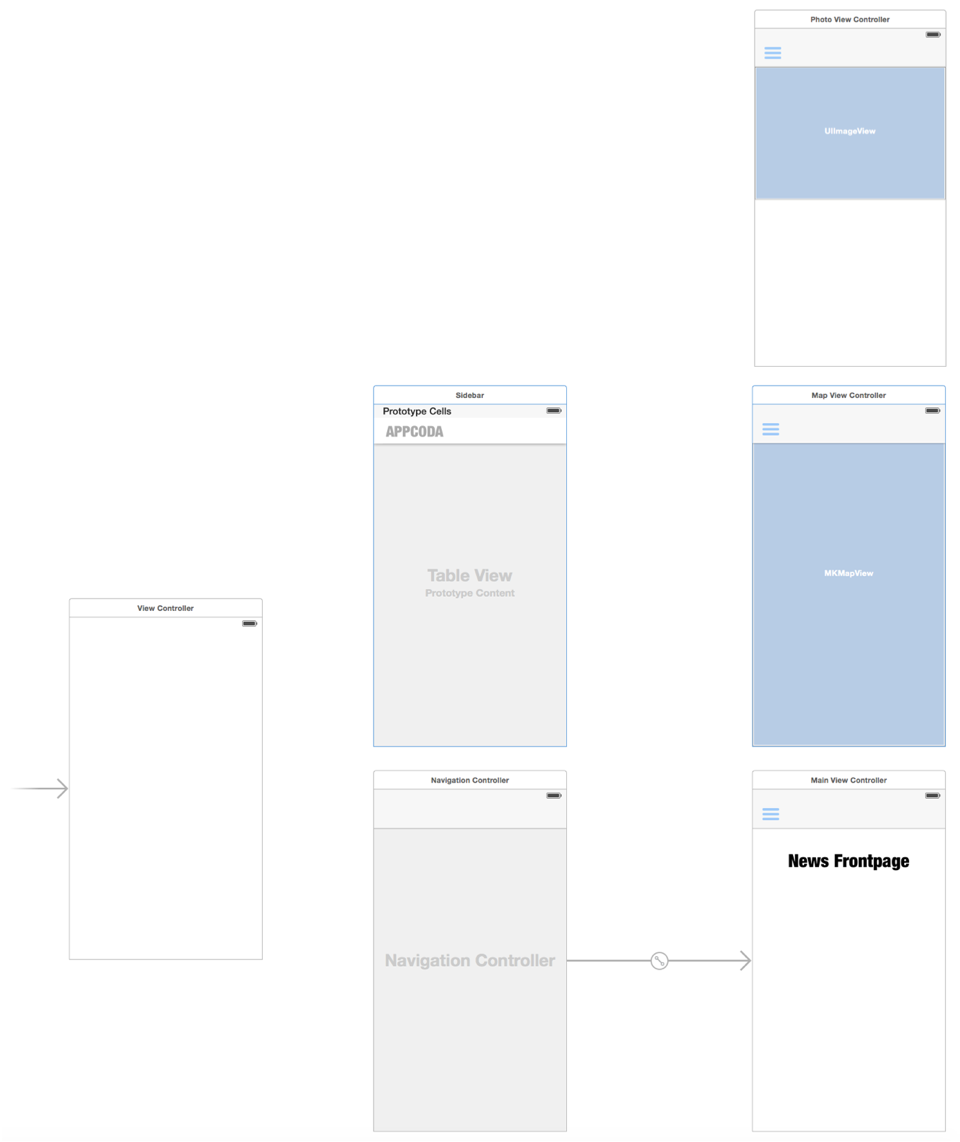
However, to save your time from setting up the project, you can download the Xcode project template to start with.
The project already comes with:
- A pre-built storyboard with all the view controllers needed
- A pre-built view controller classes including MapViewController and PhotoViewController
- The MapViewController is implemented to display a map
- The PhotoViewController is implemented to display a photo in an image view
- All icons and images needed for the app (credit: thanks for the free icon from Pixeden)
Importing the SWRevealViewController Library
As mentioned, we’ll use the free SWRevealViewController library to implement the slide-out menu. So, first download the library from GitHub and extract the zipped file.
After you extract the file, you should find “SWRevealViewController.h” and “SWRevealViewController.m”. In the project navigator, right-click SidebarDemo folder and select “New Group”. Name the group “SWRevealViewController”. Import both files into the Xcode project and put them under “SWRevealViewController”.
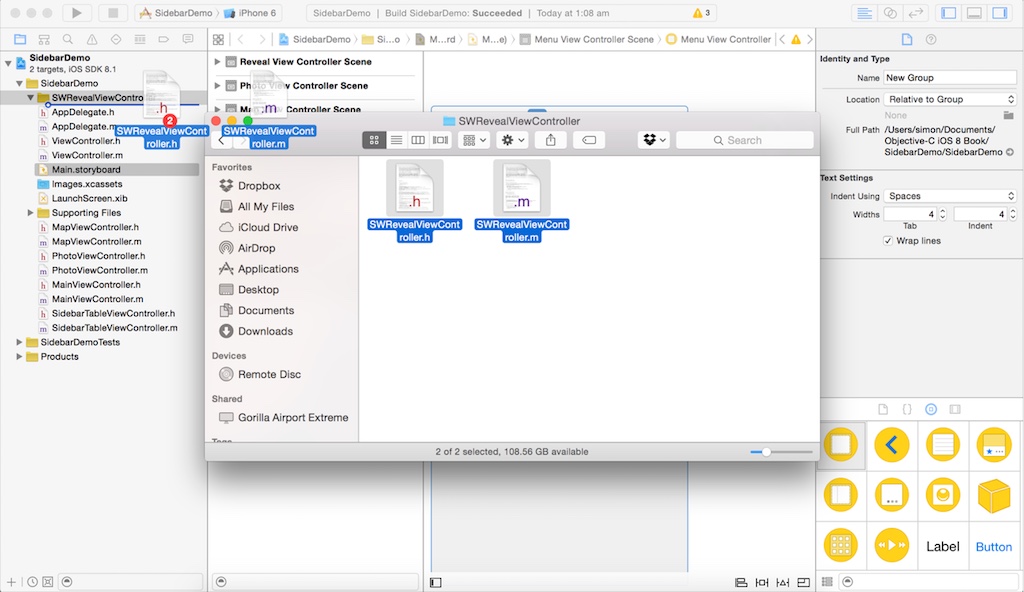
Associate the Front View and Rear View Controller
One of the beauties of the SWRevealViewController library is that it provides the built-in support of Storyboard. When implementing sidebar menu using SWRevealViewController, developers have to associate the SWRevealViewController with a front and a rear view controller using segues. The front view controller is the main controller for displaying content. In our storyboard, it’s the navigation controller which associates with another view controller for presenting news content. Apparently, the rear view controller is the controller for showing the navigation menu. Typically the menu is implemented by using UITableViewController.
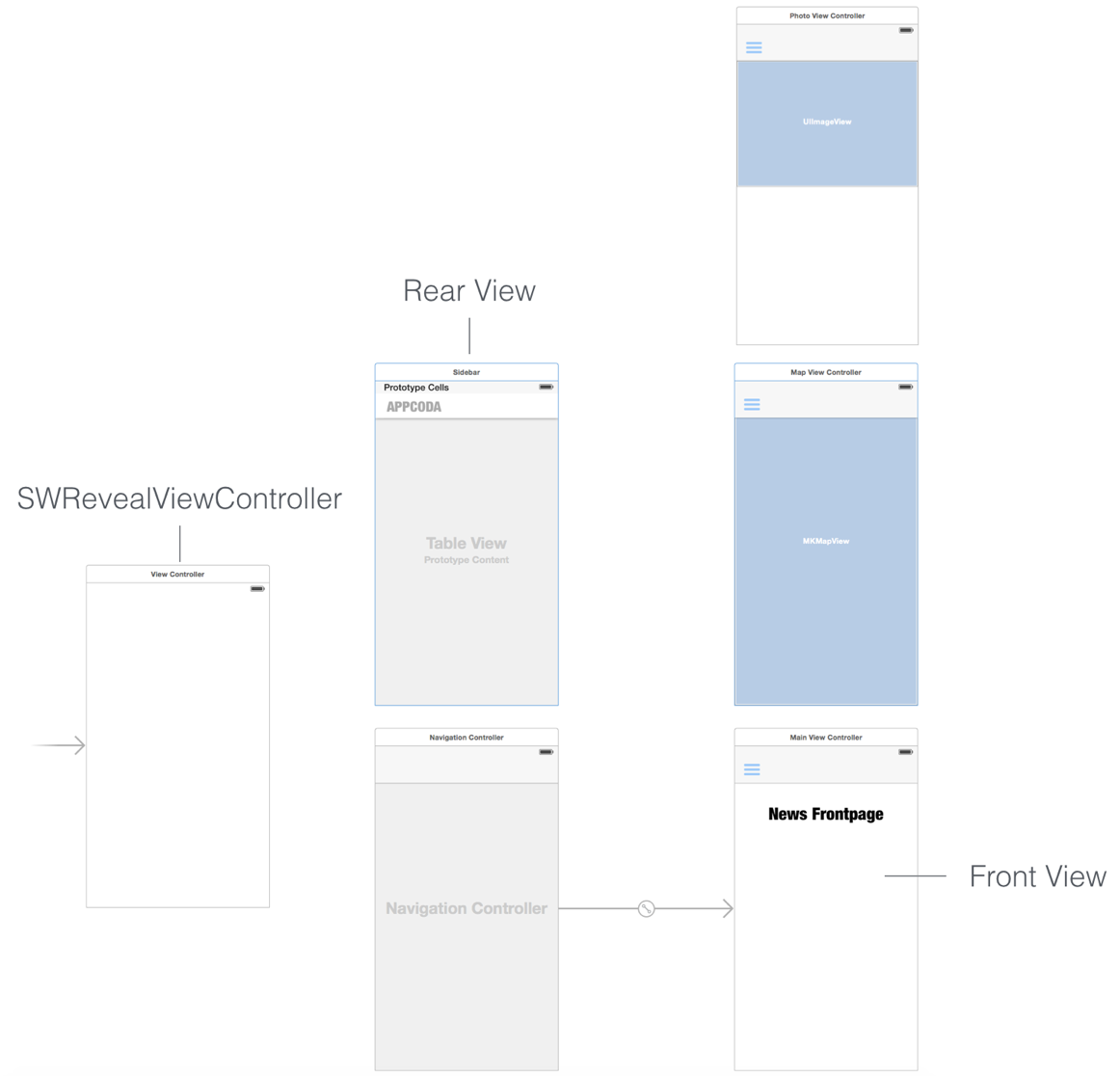
In the Xcode project template, we’ve pre-built the front and rear view controllers for you. What you have to do is to define segues between the SWRevealViewController and front/rear view controller.
First, select the initial view controller and change its class to “SWRevealViewController”.
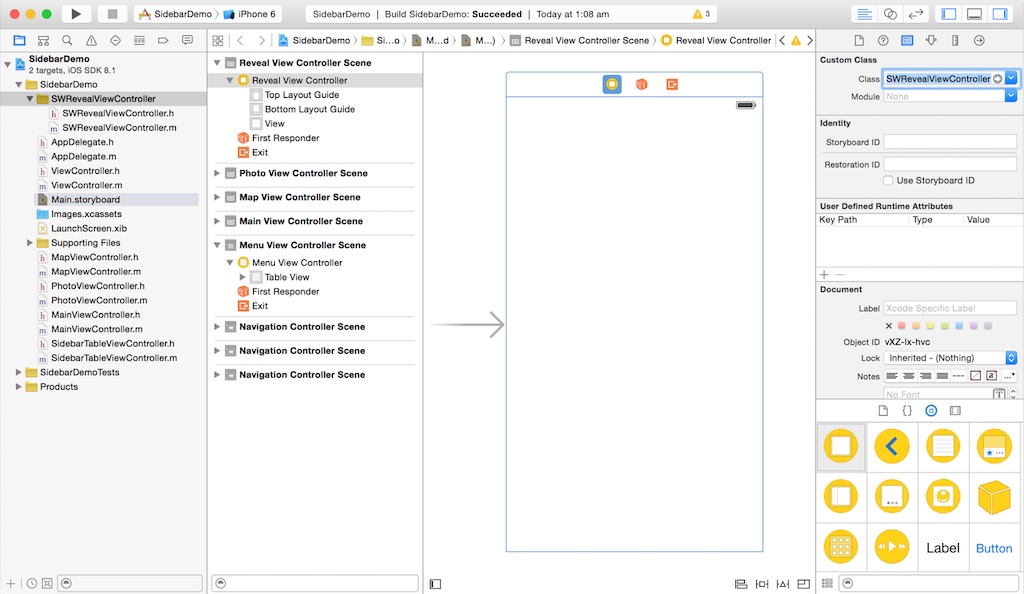
Next, press and hold the control key. Click the SWRevealViewController and drag it to the Menu view controller. After releasing the button, you’ll see a context menu for segue selection. In this case, select “reveal view controller set segue”. This defines a custom segue “SWRevealViewControllerSetSegue”. This custom segue tells SWRevealViewController that the view controller connected is the initial view controller.
Repeat the same procedures to connect SWRevealViewController with the navigation controller of the main view controller (containing News Frontpage).
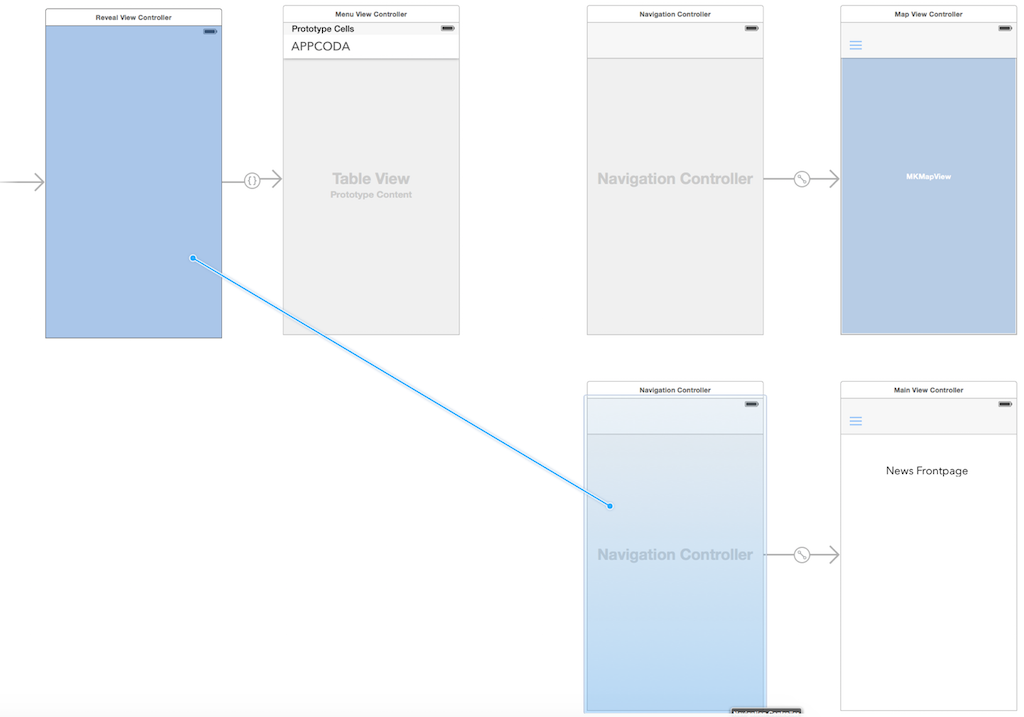
Select the segue between SWRevealViewController and the navigation controller. In the attributes inspector, set the segue identifier to “sw_front”. This is the default identifier that indicates a transition of front view controller.
For the segue between SWRevealViewController and the sidebar view controller, set the segue identifier to “sw_rear”. This identifier tells SWRevealViewController that the controller represents the rear view (i.e. the sidebar menu).
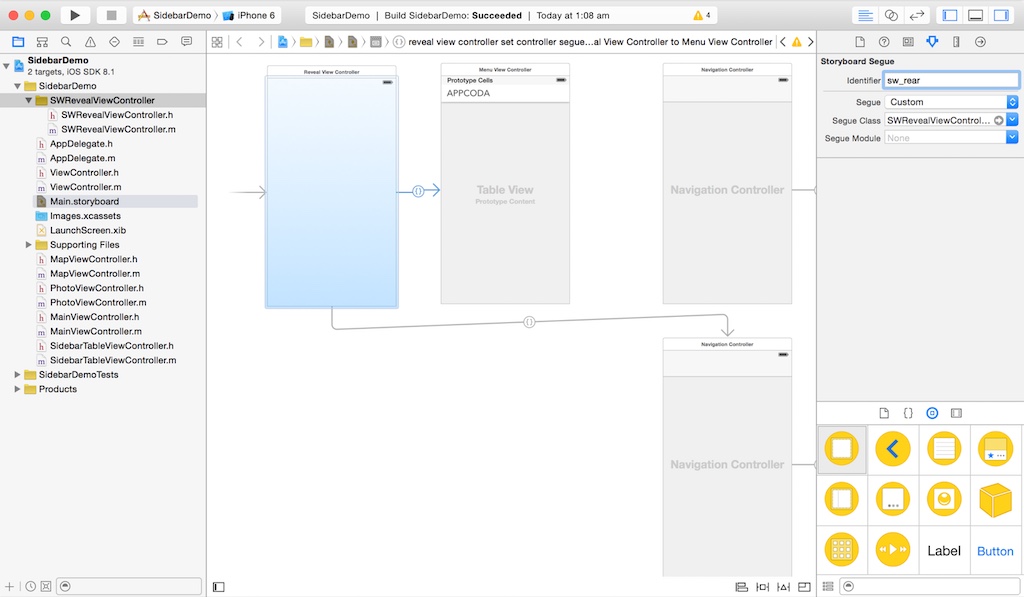
If you compile and run the app, you’ll see an app displaying the “News Frontpage”. But that’s it. You won’t see the sidebar menu when tapping the list button. We haven’t implemented the action method yet.
Open “MainViewController.m”, which is the controller class of “News Frontpage”. Add the following import statement:
1 |
#import "SWRevealViewController.h" |
Next, add the following code in the viewDidLoad: method:
1 2 3 4 5 6 7 |
SWRevealViewController *revealViewController = self.revealViewController; if ( revealViewController ) { [self.sidebarButton setTarget: self.revealViewController]; [self.sidebarButton setAction: @selector( revealToggle: )]; [self.view addGestureRecognizer:self.revealViewController.panGestureRecognizer]; } |
The SWRevealViewController provides the revealToggle: method to handle the expansion and contraction of the sidebar menu. As you know, Cocoa uses the target-action mechanism for communication between a control and another object. We set the target of the sidebar button to the reveal view controller and action to the revealToggle: method. So when the sidebar button is tapped, it’ll call the revealToggle: method to display the sidebar menu. Lastly, we also add a gesture recognizer. Not only you can use the list button to bring out the sidebar menu, user can swipe the content area to activate the sidebar.
Try to compile and run the app in the iPhone simulator. Tap the list button and the sidebar menu should appear. Tap the button again to close it. You can also swipe right on the content area to open the menu just like the figure below.
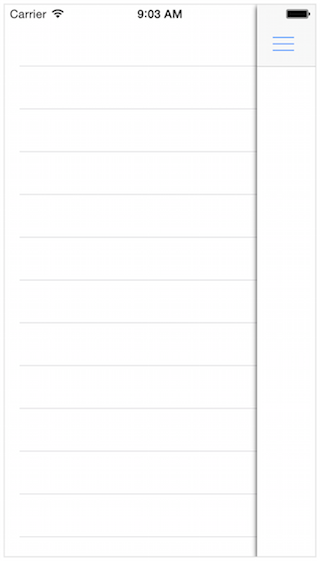
Before moving on, add the same code snippet in the viewDidLoad: method of both PhotoViewController.m and MapViewController.m. The app should show up the sidebar when a user taps the list button in these two view controllers.
Adding the Menu Items in Navigation Menu
With just a few lines of code, you already implement the slide-out navigation menu. Cool, right?
However, the menu is now empty. We’ll now add a few items and show you the transition from one item to another. The sidebar view controller is just a simple table view controller. For sake of simplicity, we’ll design the sidebar menu right in the storyboard.
The first table cell of the Sidebar View Controller is defined with the title “APPCODA”. If you don’t like it, just change it to whatever you prefer. The only thing you have to ensure is to keep the cell identifier as “title”, which we’ll refer it later in our code.
Okay, let’s add a few more menu items. To begin, select the prototype cell and change the number of prototype cells to 8 in the attributes inspector. You should end up with a screenshot similar to below:
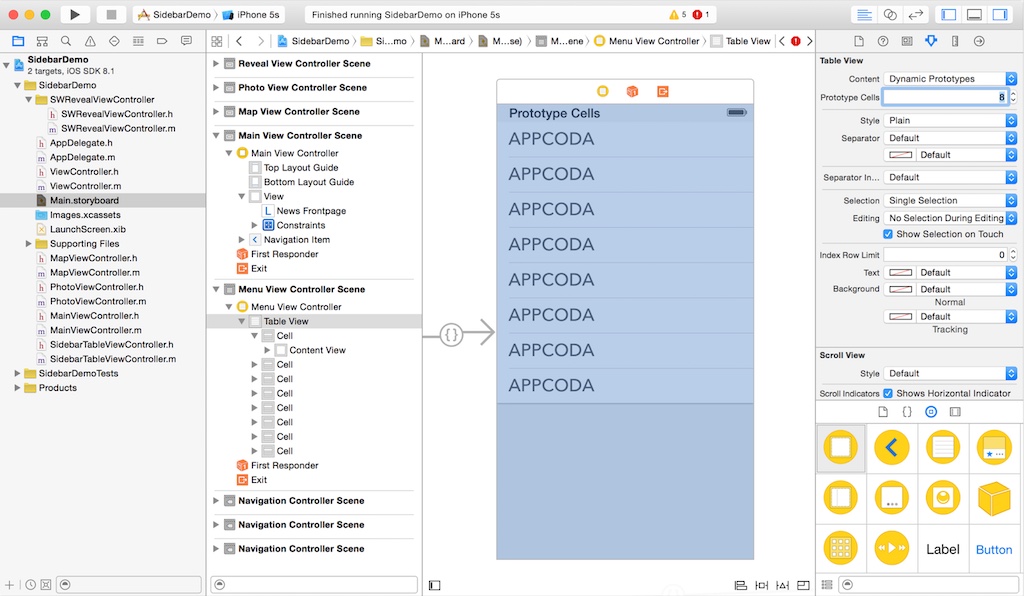
Change the “APPCODA” label of the second cell to “News”. Optionally, you can change the color of label to dark gray and set the font to “Avenir Next” in the attributes inspector. Next, drag a image view object from the object library to the cell. Set the size of image view to 38×38 and change the image to “news.png”.
Next, select the cell and set the cell identifier as “news” in the attributes inspector. You should end up with a cell similar to the screenshot below.
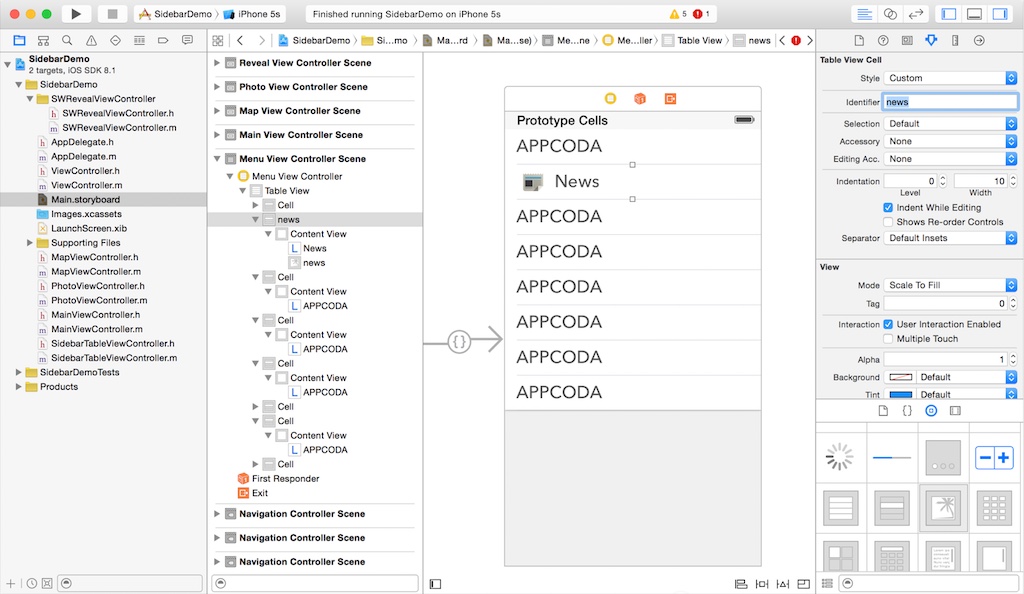
Repeat the above procedures to add the following menu items:
- Comments (set the images as comments.png and cell identifier as comments)
- Map (set the images as map.png and cell identifier as map)
- Calendar (set the images as calendar.png and cell identifier as calendar)
- Wishlist (set the images as wishlist.png and cell identifier as wishlist)
- Bookmark (set the images as bookmark.png and cell identifier as bookmark)
- Tag (set the images as tag.png and cell identifier as tag)
If you’ve done everything correct, your sidebar view controller should look like this:
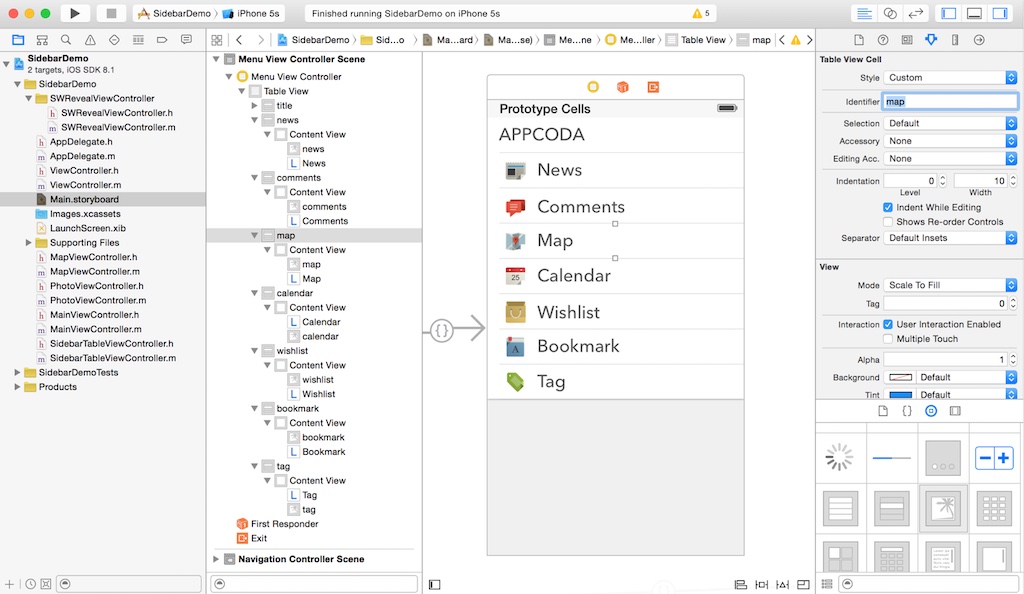
After completing the user interface, let’s implement the code for displaying the table cell. Select the “SidebarViewController.m” and add the following import statement:
1 |
#import "SWRevealViewController.h" |
Next, declare the menuItems variable to store the cell identifier of the menu items:
1 2 3 |
@implementation SidebarViewController { NSArray *menuItems; } |
Update the viewDidLoad: method to the following:
1 2 3 4 5 6 |
- (void)viewDidLoad { [super viewDidLoad]; menuItems = @[@"title", @"news", @"comments", @"map", @"calendar", @"wishlist", @"bookmark", @"tag"]; } |
The above code is very straightforward. We simply initialize the menu item array with the values of cell identifier. Then edit the numberOfSectionsInTableView: method to return 1 and the numberOfRowsInSection: method to return the correct number of table row:
1 2 3 4 5 6 7 8 9 |
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView { // Return the number of sections. return 1; } - (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section { // Return the number of rows in the section. return menuItems.count; } |
Lastly, change the cellForRowAtIndexPath: method to the following:
1 2 3 4 5 6 |
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath { NSString *CellIdentifier = [menuItems objectAtIndex:indexPath.row]; UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath]; return cell; } |
The code simply gets the cell identifier of the specified table cell from the menuItems array for display. Now compile and run the app again. Tap the list button and you’ll find a slide-out navigation menu with menu items.
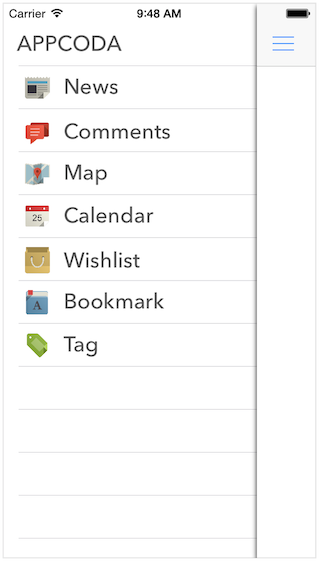
Implementing Menu Item Selection
You’ve already built a visually appealing sidebar menu. There is still one thing left. For now, we haven’t defined any segues for the menu item. When you select any of the menu items, it will not transit to the corresponding view.
To keep the demo app simple, we’ll only connect the menu item with three view controllers. I think this should give a pretty good demonstration to show you how it works. Here are what we’re going to do:
- Connect the “News” cell item with the main view controller using a “reveal view controller push controller” segue
- Connect the “Map” cell with the map view controller using a “reveal view controller push controller” segue
- For the rest of the menu items, they will be associated with the photo view controller using the same type of segue. But we’ll display different photos for different menu items.
Okay, go back to storyboard. First, select the map cell. Press and hold the control key and click on the map cell. Drag to the navigation controller of the map view controller and select the “reveal view controller push controller” segue under Selection Segue.
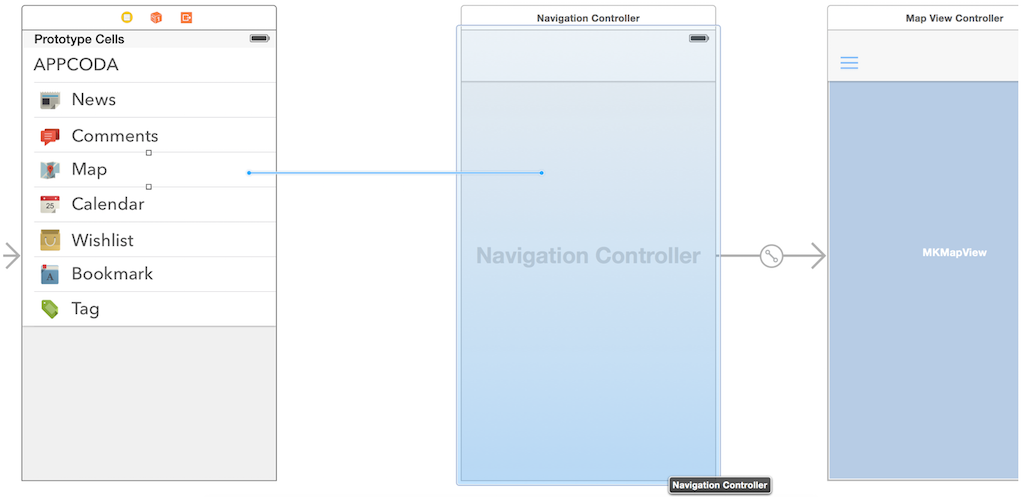
Repeat the above procedure for the “News” cell item, but connect it with the navigation controller of the main view controller.
For the rest of menu items including comments, calendar, wishlist, bookmark and tag, connect them one by one with the navigation controller of the photo view controller and set the segue identifier as “showPhoto”. We’ll use this identifier to differentiate the segue from the previous two we created.
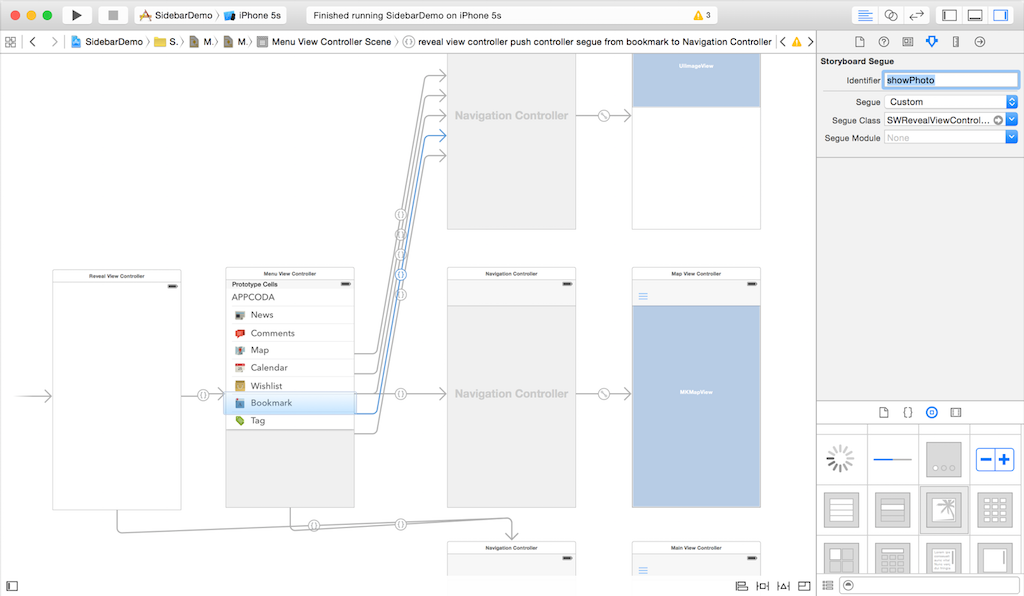
Once you complete the configuration of segues in storyboard, open the “SidebarViewController.m”. First add the following import statement:
1 |
#import "PhotoViewController.h" |
Then insert the prepareForSegue: method to manage the transition.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender { // Set the title of navigation bar by using the menu items NSIndexPath *indexPath = [self.tableView indexPathForSelectedRow]; UINavigationController *destViewController = (UINavigationController*)segue.destinationViewController; destViewController.title = [[menuItems objectAtIndex:indexPath.row] capitalizedString]; // Set the photo if it navigates to the PhotoView if ([segue.identifier isEqualToString:@"showPhoto"]) { UINavigationController *navController = segue.destinationViewController; PhotoViewController *photoController = [navController childViewControllers].firstObject; NSString *photoFilename = [NSString stringWithFormat:@"%@_photo", [menuItems objectAtIndex:indexPath.row]]; photoController.photoFilename = photoFilename; } } |
In the above code, we first retrieve the current cell identifier and set it as the title of the navigation bar. For those segues with “showPhoto” identifier, the photo view controller will only display a single photo. Here we set the file name of the photo to be displayed. Say, when user taps the “Comments” item, we’ll show the “comments_photo.jpg”.
Compile and Test the Final App
Now compile and test the app again. Open the sidebar menu and tap the map item. You’ll be brought to the map view. Try to test other menu items and see what you get.
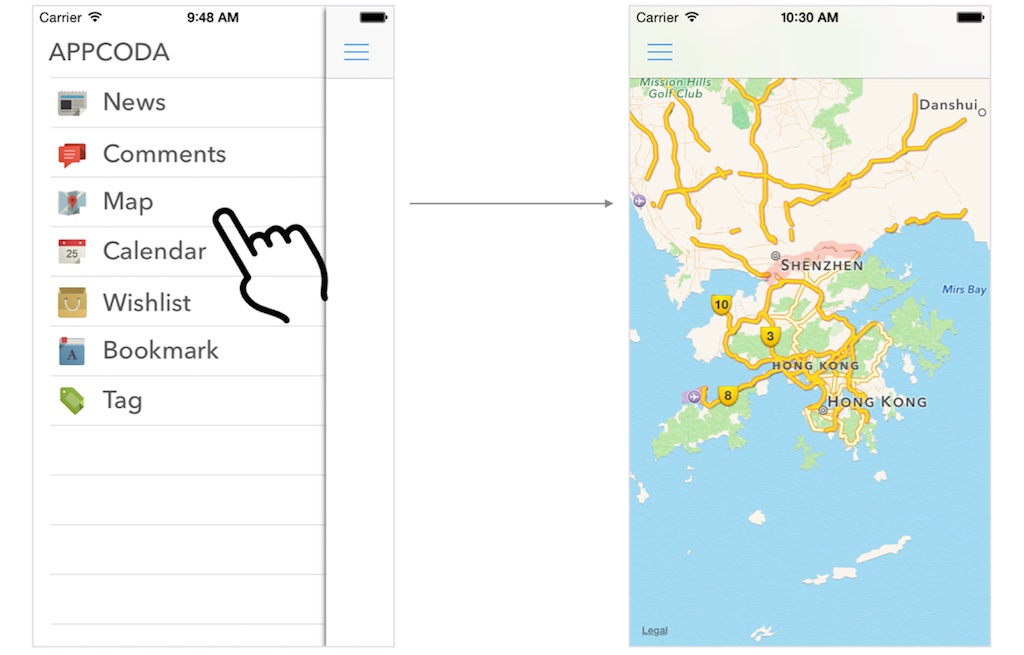
Summary
In this tutorial, we show you how to apply SWRevealViewController to implement a slide-out navigation menu similar to the one in the Facebook app. If you do a search in GitHub, you can find other sidebar solutions such as GHSidebarNav and ViewDeck. You’re free to explore other libraries and see which is the best fit for your app. If you’d like to learn how to build the sidebar menu from scratch, Ray Wenderlich offers a great tutorial.
For your complete reference, you can download the full source code from GitHub. As always, leave us comment and share your thought about the tutorial.
Note: If you’re still using Xcode 5, you can download the project from here.
Comments
napolux
AuthorAwesome! I used the component in some previous project and it’s really easy to implement. Your tutorial covers the “lack” of a good example on the project site.
Thanks!
Joseph
AuthorHey,
Great tutorial, will this be able to work in iOS 7?
Simon Ng
AuthorNot tested on iOS 7 yet, but I think it should work. Anyway, I’ll do a test on iOS 7 beta 2 later.
Moritz
AuthorHey, awesome work.
I am a fan of this page for over six month.
I didn’t get it working with iOS7 and XCode5. Had someone tried it and can share their experience?
bsoriano
Authorif ios7 works but when I add another navigationController I get error when invoking it from the tableviewcell, know how to fix this
Rick
AuthorDoes not work in iOS 7… Any plans on posting a comment on how to get it to work in iOS7? Someone said use UIViewController, drag a tableview, add some delegate and add some property… While this could be obvious for experts I think articles here are targeted at novice/intermediate devs… Would it be possible at all to update or post a comment detailing how to achieve that please?
macuser79
AuthorFantastic … I wanted a tutorial that would explain how to perform this type of implementation … Thanks a lot
Simon Ng
AuthorYes, the sidebar view controller is actually a UITableViewController. So you can render it dynamically using your own method.
macuser79
AuthorOk thanks……
vat
AuthorURGENT: When I do all of the steps up to just getting the menu to show up, when I compile and run it says in the console “Unknow class SWRevealController in Interface Builder File”? Any help please. 🙁
Simon Ng
AuthorIs it a typo? The class should be “SWRevealViewController” instead of “SWRevealController”.
Rod
AuthorHey, just select the SWRevealViewController.m class and then go to View > Utilities > Show File Inspector.
Make sure your project is checked under Target Membership.
Gabriel
AuthorWhat do you mean about Target Membership?
Gabriel
Authorok i found it,thanks
Joel
AuthorHad the same error and the solution for me was:
1) Select your Project in the Sidebar
2) Go to “Build Phases”
3) Make sure SWRevealcontroller.m is listed in the “Compile Sources” list
4) Build and Run
abc
AuthorIt’s still not working for me ? Any other suggestions ?
Ioannis
Authorgreat work as always!very glad i found this site a couple of months ago. still a newbie but helped me learn many many things:)keep up the good work!!
Rafael Avila
AuthorAwesome tutorial, thank you so much!
Harish Geeth
AuthorHey, awesome tutorial. I like SWRevealViewController better than ECSlidingViewController. Very easy to use and less code. One question I have is,
I have a LogInViewController -> NewsFeed. While authenticating user, I am sending some information to the NewsFeedViewController. How do I handle that type of situation ?
Your thoughts are highly appreciated.
Thank you.
pascal
AuthorThis is insanely great stuff that you guys are providing with each one of your posts – really appreciate this.
LittlePeculiar
AuthorAs always, well done. I love the little tricks I learn from your tutorials.
Thanks
Ben Azoulay
AuthorUrgent please how to add a sidebar button in navigationcontoller?????
Simon Ng
AuthorIn Storyboard, just drag a Bar Button Item from the Object library to the navigation bar to add the sidebar button.
Alaeddine Ghribi
AuthorAnd what about adding a button into the NOT ROOT ViewController navigation bar?
When opening a view controller from the side menu bar, I have automatically the navigation bar, but can’t add anything on it because it’s not present in the StoryBoard.
Ben Azoulay
AuthorI have the same thing…..
Alaeddine Ghribi
AuthorOkay, here is what I did right now, just hide the automatic navigation bar with: [self.navigationController setNavigationBarHidden:YES]; Then add a navigation bar from the storyboard and add buttons. Don’t forget to add [self.navigationController setNavigationBarHidden:NO]; in the root controller.
Alaeddine Ghribi
AuthorThank your for your tutorial. I’m facing a problem : when I tap on any element on the UITableView sidebar, nothing happens. The segue identifiers are pretty ready and configured. Any hints please?
Alaeddine Ghribi
AuthorCan I add different (more than ONE) side bars for different view controllers?
Elohim
AuthorYes you just have to add another UITableView, connect it as the first one but assign its segue as sw_right, instead of sw_rear. And in the .m file of the class tht manage the ViewController where you wanna have 2 menus..in the viewDidLoad method, set the .action of the button as @selector(rightRevealToggle:); instead of @selector(revealToggle:);
Souhail Marghabi
AuthorHi, I enjoyed reading your tutorial. i would like to ask for help about the following problem: In my app, i have several view each displaying and doing something in core data, and for now my rootviewcontroller is a tableviewcontroller embedded in a nav and I wanted to know what are the changes i need to do in the rootviewcontroller or app delegate.m to add the sidebar visible in every view. My rootviewcontroller is implemented the same way as this : http://developer.apple.com/library/ios/#samplecode/CoreDataBooks/Introduction/Intro.html
Mark Crippen
AuthorHey, i have followed the tutorial correctly, however i keep getting a weird error
0x22ab94d: calll 0x22ab952 ; CA::Layer::sublayers() + 14 Thread 1: EXC_BAD_ ACCESS(Code=2 Address=0xbf7fffec)
I get this error whenever i click on the tableview to segue to a new Navigation Controller. what would cause this? how can i fix it?
Mark Crippen
AuthorI fixed my own error. I had the Segue going to the Navigation Controller and not to the actual View Controller.
bsoriano
Authorwhen I add viewcontroller if it works but if I want to add another navigationController get the same errror, it came to solving
Max
AuthorHello, i have follow this great tutorial, but i have a question. I have a TableViewController with an NSArray and a view controller. I want all items of my table view opens my ViewController.
Help please it’s urgent.
Sorry for my bad english i’m french.
Jacob Klapper
AuthorThank you very much, this was very helpful! I have a question though: How do I render a cell in the table “unusable” or grayed out? Like let’s say I press on the tags cell, now I want that cell to be grayed out next time I open the menu, how do I do that?
Thanks
ilikepear
AuthorTested it on iOS7 beta2.. indeed broken, so is many sidebarnav’s like GHSidebarNav
Looks on simulator and device(ip4) as per below.. slightly different but still broken.
Guest
Authorwhere you able to resolve this for ios 7 beta
Gunnar
AuthorA temporary fix: Add an image view on top of the table view and adjust the height of the image so it suits your needs.
joelypolly
AuthorProper fix is to put a view outside the UITableView and change the UITableViewController to a UIViewController and add UITableViewDataSource, UITableViewDelegate
hanmookwan
AuthorAdd image view – height 20
Guru
Authorcan we implement for ipad?? with same swrevealviewcontroller
Rakari
AuthorI found this on stack overflow: – (void)tableView:(UITableView *)tableView willDisplayCell:(UITableViewCell *)cell forRowAtIndexPath:(NSIndexPath *)indexPath{
[cell setBackgroundColor:[UIColor colorWithWhite:0.2f alpha:1.0f]];
} Just put it in the SidebarViewController.m
Canard
AuthorThanks !
It works perfectly !
Jacob Klapper
AuthorAfter completing this tutorial, if I try to add new cells to the tabelview, the height/width of the new label is unchangeable, any idea why?
GeekBrony
AuthorIt’s probably constraints locking the two variables.
Souhail Marghabi
AuthorCan You do a tutorial on sidebar with one of the apps in your other tutorials that has views with functionalites rather than display?
Souhail Marghabi
Authorhi, Can you tell us what to do when we want to integrate this to an existing app?
saad maghrabi
AuthorIf the app doesn’t use Storyboard, just pick the sources SWRevealView, add them; then connect them to your project.
Souhail Marghabi
AuthorAnd with an already existing storyboard? what do i need to change in the app.
benjones73
AuthorWhen I drag the news or other image in to each cell after changing the label and font. It comes in huge and I am wondering how to resize it to the correct size.
Joel
AuthorDid you insert it into an image view of the correct size?
asinox
AuthorGreat!, thank you
Robert
AuthorGreat, tutorial.
However how could I make this so the menu is on the right?
Elohim
AuthorAssign its segue as sw_right, instead of sw_rear. And in the .m
file of the class in the viewDidLoad method, set the .action of the button as
@selector(rightRevealToggle:); instead of @selector(revealToggle:);
Pepper
AuthorI’m trying to add state preservation & restore to this app. Since it only uses storyboards, from what I’ve found online, I should only need to implement methods and not set up a class:
– (BOOL)application:(UIApplication *)application shouldSaveApplicationState:(NSCoder *)coder
{
return YES;
}
– (BOOL)application:(UIApplication *)application shouldRestoreApplicationState:(NSCoder *)coder
{
return YES;
}
in my app delegate and then set up a unique restoration id for every controller (including the reveal view controller & navigation controller). When this didn’t work, I then set up a unique restoration id for every table view and view in the storyboard as well. Still no luck. Any suggestions? Thanks!
Joao Paulo
AuthorHello, great tutorial, many thanks for that.
But, what if I have another ViewController as my initial view controller?
I’ve got a logoViewController(Splash View) as my initial one.
I tried to compile following the tutorial and I got:
*** Terminating app due to uncaught exception ‘NSInvalidArgumentException’, reason: ‘*** -[__NSArrayM insertObject:atIndex:]: object cannot be nil’
Fibla
AuthorI have the same problem. You found the solution?
Joao Paulo
AuthorNope, I tried a couple of other ways of doing a Slide Menu, but no success as well. I will give it a go again with this one and let you know.
Joel
AuthorIt would be really helpful if you’d have highlighted the “Before moving on, add the same code snippet to both PhotoViewController.m and MapViewController.m.”. I didn’t see it the first time when I’ve read the tutorial, otherwise great tutorial!
rgb
Authorgreat tutorial. simple question, i have a tab bar style app that i am converting to this (slide view) style menu, each tab is its own navigation controller and everything works fine. on conversion, how can i segue from the menu items to a new navigation controller? your tutorial re-uses the navigation replacing the original view controller with the destination view controller. thanks.
Jeremy Langer
AuthorI was having this same issue. Here’s what I did to solve it:
1. Give each navigation controller you want to be included in the menu a Storyboard ID (in the Identity inspector), such as “navTest”.
2. Create a SWRevealViewControllerSegue from each row of the menu to its navigation controller. Give each segue a unique identifier, such as “showNavTest”.
3. Go to the View Controller for your slide out menu and go to your prepareForSegue.
– (void) prepareForSegue: (UIStoryboardSegue *) segue sender: (id) sender
{
// Make sure we are using the SWRevealViewControllerSegue
if ( [segue isKindOfClass: [SWRevealViewControllerSegue class]] )
{
SWRevealViewControllerSegue *swSegue = (SWRevealViewControllerSegue*) segue;
swSegue.performBlock = ^(SWRevealViewControllerSegue* rvc_segue, UIViewController* svc, UIViewController* dvc)
{
// The name of our view controller we want to navigate to
NSString *vcName = @””;
// Set the name of the Storyboard ID we want to switch to
if ([segue.identifier isEqualToString:@”showNavTest”])
{
vcName = @”navTest”;
}
else if ([segue.identifier isEqualToString:@”showNavOtherTab”])
{
vcName = @”navOtherTab”;
}
// If we selected a menu item
if (vcName.length > 0)
{
// Get the view controller
UIViewController *vcNew = [[UIStoryboard storyboardWithName:@”MainStoryboard_iPhone” bundle:NULL] instantiateViewControllerWithIdentifier:vcName];
// Swap out the Front view controller and display
[self.revealViewController setFrontViewController:vcNew];
[self.revealViewController setFrontViewPosition: FrontViewPositionLeft animated: YES];
}
else
{
// Could not navigate to view – add logging
}
};
}
}
Jeremy Langer
AuthorHere’s a screenshot for easy readability:
Ben Azoulay
Authori have this issue: return UIApplicationMain(argc, argv, nil, NSStringFromClass([AppDelegate class]));
when i try this. please help
bsoriano
Authorexcellent tutorial, I’m using it but when I want to add another navigationcontroller for a listing RSS, I get the same error … any other suggestions
Rakari
AuthorYou have probably messed up the connections in the storyboard or something. does’nt the error say something about SIGABRT?
bsoriano
Authorexcellent tutorial, I’m using it but when I want to add another navigationcontroller for a listing RSS, I get the same error what Ben … any other suggestions
misato
AuthorHi Jeremy,
I had the same issue but thanks to your tips, I got it to work. Thanks!
Ulix
Author1.
In the SidebarViewController the identity is SidebarController, bur “Use Storyboard ID” is uncheck
Other Viewcontroller (eg PhotoViewController MapViewController….) need the same IDStoryboard (navtest) and Use Storyboard uncheck? and Restoration ID is not necessary?
2.
I have 20 rows in the sidebar menu, How and where should I set the SWRevealViewControllerSegue?
3.
in which ViewController i put the code? in each?
thanks for the answers
Gavin Carter
AuthorI simply made sure each view controller had the following coded into its .m file:
// Set the side bar button action. When it’s tapped, it’ll show up the sidebar.
_sidebarButton.target = self.revealViewController;
_sidebarButton.action = @selector(revealToggle:);
// Set the gesture
[self.view addGestureRecognizer:self.revealViewController.panGestureRecognizer];
Make sure that you also import the relevant .h file:
#import “SWRevealViewController.h”
Behold, back navigation from view controllers working fine! Everything you need above can be copied out of your MainViewController.m file
Infinite∞Development
AuthorHELPPPP I got everything working but the
// Set the gesture
[self.view addGestureRecognizer:self.revealViewController.panGestureRec
The error read ‘panGestureRec’ not found on object of type ‘SWRevealViewController*’ Any suggestions?
Nathaniel Lee
AuthorRec is a typo, its recognizer
Jennifer
AuthorThis is the worst tutorial I’ve ever used. The code just didn’t work and now I’ve wasted so much time trying to learn something that just didn’t work.
george
AuthorHELP…. when i open a tab which isnt news, the bar button item goes blue in color and im not able to open the side nav anymore?
Rakari
AuthorSame issue here
Rakari
AuthorFound out! you just copy the lines _sidebarButton.target = self.revealViewController;
_sidebarButton.action = @selector(revealToggle:); in the MainController
to both MapViewController and PhotoViewController
Lu
AuthorDon’t forget this line as well: [self.view addGestureRecognizer:self.revealViewController.panGestureRecognizer];
Guest
Authorsegue is going from slide out menu items to UIViewControllers.
there come two options selection segue or accessory action
Usman Ahmad
Authorsegue is not going to the UIViewController from slide out menu items.
there are coming 2 options. 1 is segue action other is accessory option. check my attached image
Nathaniel Lee
AuthorDo selection, reveal view controller
Usman Ahmad
Authorthanks buddy. it is solved.
John
AuthorNice Tutorial. helps me a lot 🙂
npersson
AuthorDoes this work on iOS 5 or 5.1?
Juan Luis
AuthorIm not able to use it in ios7 beta 6, any suggestion?.it brokes when i write this line in viewDidLoad
[self.view addGestureRecognizer:self.revealViewController.panGestureRecognizer];
any suggestion? thanks.
Ludwig Paulsen
AuthorI followed the tutorial step by step and get those errors on the last step any suggestions? (XCode 5 DP beta)
In the prepareForSegue method the last paragraph (the transitions) causes several issues. “Use of undeclared identifier SWRevealViewControllerSegue”
“Use of undeclared identifier “swSegue”, did you mean “segue”? and 13 other errors. 😮
Nathaniel Lee
AuthorI have a quick workaround for this on IOS 7,
What you do is when you set up the sidebar controller, instead of dragging in a table view controller, you put a regular view controller and drag a table view into it. This allows you to resize the table view to accommodate the menu bar.
In your code, all you have to do is add next to the interface declaration, and add a @property for the object, tableView
Now with the dark gray color change, you have to do that in interface builder (at least thats how I did it)
Usman Ahmad
Authorworked. its awesome tutorial
shiva kumar
Authorwhat is the options and bars in xcode 4.X&4.x
Ulix
AuthorThe tutorial is very good, but I have 2 problems
First problem: with Xcode 5 Beta (iOS7) the App work fine but the Menu Button is not visible
Second problem: in this MapViewController I would like the map is opened with a specific address, and with the ability to create a route (with car) It’s possible?
Rakari
AuthorAnswer to first problem: comment out the line in MainViewController that says _sidebarButton.tintColor = [UIColor colorWithWhite:0.96f alpha:0.2f];
Answer to second problem: Go into the mapViewController and watch this tutorial https://www.youtube.com/watch?v=fvj0ywGUM24 (sorry it’s xcode 4)
george
AuthorHi im new to app dev,
how do i add pages to the demo app, what i want is about 8 of the main view controllers (just blank pages )with the sidebar icon at the top to make different pages in the app. I have tried to copy the code but all that comes back when i make a new file and paste the code is _sidebar error please help
bob
Authorcan anyone make a video tutorial of how to put it all together including adding new pages!
Guest
AuthorAsk how to fix error code …
hanmookwan
AuthorAsk how to fix error code …’multiful segues wiht identifier showPhoto’
Emil Tinebo
AuthorThis is a really great tutorial. I downloaded your Xcode Project Template and followed the tutorial. Worked out fine. What I want to do now is that I want to change the background color of the navigation bar but I only manage to set the background color of the sidebarButton. Can anyone point me in the right direction of how to accomplish this?
Rakari
Authorgo to the navigation controller and select the navigation bar and change the bar tint to whatever you want
hanmookwan
Authorcreated a new ViewController.
Navigation Bar comes out abnormally. Please tell me what is the problem.
-ViewController.m-
#import “TagViewController.h”
#import “SWRevealViewController.h”
@interface TagViewController ()
@end
@implementation TagViewController
– (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
– (void)viewDidLoad
{
[super viewDidLoad];
_sidebarButton.target = self.revealViewController;
_sidebarButton.action = @selector(revealToggle:);
_sidebarButton.tintColor = [UIColor colorWithWhite:0.96f alpha:0.2f];
}
– (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
Paul
AuthorSo I can get the side menu to work properly on my first view controller called “view controller” in the image. However, I need to be able to load the first view controller (it loads necessary data for classmates view controller in the image i have provided), and then segue to my classmates view controller, and have that access the side menu. So the view controller should not have access to the side menu, but once I have segued into my classmates view controller, that should. I cannot seem to implement this, I think it has something to do with the reveal controller needing to be the initial view controller. I need my “view controller” to be the initial one, and the side menu to be accessible from my classmates view controller. Essentially, the first page is a login page, and doesnt need the side menu, until the user is logged in. Any ideas?
Chelsea
AuthorYou might have figured this out by now, but I thought I would go ahead and add in a comment just in case you haven’t or someone else needed the solution as well. All you would need to do in this case is set the login page as your root view controller, and instead of having a segue from that to you classmates view controller, simply have it segue to the SWRevealViewController which has the classmates view controller as it’s sw_front. I have a similar thing working on my app so it should work if I explained it correctly. =)
puneet
Authorhey…i tried that but its giving me a back button instead of my reveal icon, i tried hiding it from swreveal view controller but then it doesnt show me my reveal icon
Becki
AuthorI am having the same issue, did you manage to fix this?
Julien Tupula
AuthorHi Becki did you manage to fix this issue?
Shikhar Singhal
Authorhave a look at this link-
http://stackoverflow.com/questions/34287457/xcode-sidemenu-with-swrevealviewcontroller/34329739#34329739
Akanksha Saxena
AuthorHey I still could not find a solution to this problem. Its not working if I keep my login page as the initial view controller. Kindly help.
Hossein
AuthorGreat tutorial really , thanks for that , i have a question , is there a way the menu open from right to left ?
i mean reverse operation that in this tutorial say….in fact i want the menu button , go to the right of navigation control and the menu open from right , now it’s open from left in this tutorial… is there a way for this?
Diana Sutandie
AuthorOpen the .h file, they have provided some implementations for reversed version. You have to changed the sw_rear into sw_right as well.
Leo
Author@selector(rightRevealToggle:);
Jerry Salazar
Authorhi, i have one problem, when i move to other View i lost the button back, and i want that show the button, ok thanks, great tutorial, but you do have a solution for this?
lavo20
AuthorI followed the tutorial exactly and I get “Scene is unreachable due to lack of entry points and does not have an identifier for runtime access via -instantiate ViewControllerWithIdentifier:” Why is this?
hanmookwan
AuthorCreated a new ViewController.
i don’t create navigation bar.
– navigation bar + item (?) Does this create?
I want to create a Navigation bar is known.
guest
AuthorI guess you’re japanese ?
Benjamin Porter
AuthorGreat tutorial! Except when I run it there is a black bar on the top that I can’t seem to get rid of. Also when you slide down on the menu, a spinning wheel comes up and it wont go away. Any suggetions?
AnthonyMarchenko
AuthorFor make status bar white. Add two property to the .plist file
UIStatusBarStyleLightContent (String) – UIStatusBarStyleLightContent
View controller-based status bar appearance (bool) to NO
Jeromii UnReal Anderson
Authori want to excuete this tutorial but by using XIB can somebody help me please.
VansFannel
AuthorAnd, if we are not using Storyboards?
dan
Authori get an error code when i try to open the sidebar menu. It stops the simmulator and brings up this code:
@autoreleasepool {
return UIApplicationMain(argc, argv, nil, NSStringFromClass([AppDelegate class]));
}
}
thread 1: signal SIGABRT.
Rakari
AuthorThen there’s something wrong connected in the storyboard
Milan Skrecek
AuthorGreat tut. But I couldn’t get it to work. Did anyone really get this slideout menu working for Xcode5? If so, it there a link to the final source files?
Brain
AuthorHello, it is a great tutorial and i used it to develop an app, but when i updated the ios7, the image of menu button was invisible. Could you help me solve this?Thanks a lot!
Diego Giuliani
Authorme too
same problem
Bianca Araujo
Authorto fix this problem:
comment out _sidebarButton.tintColor = [UIColor colorWithWhite:0.96f alpha:0.2f];
Sanchit Choubey
Authorexcellent Demo…:) thanks..!!
bsoriano
Authoryou can place a submenu for each item, similar to the current app of the university of texas
張璋
Authorexcuse me, do you know how to control how long we slide. in other words, can i control the size of the sliding menu? thank you
Erv
AuthorI am doing this on xcode 5 and I keep getting the warnings: “Misplaced View – Frame for ‘Label News’ will be different at run time.” What am I doing wrong ?
abhi
Authorthe navigation bar disappears when i switch the views
Svetlin Stoev
AuthorThe problem with the black bar at the top is because of iOS7 new status bar. More about this can see here http://www.doubleencore.com/2013/09/developers-guide-to-the-ios-7-status-bar/
Drake Grossman
Authorwill this work with tab bar item instead of bar button item?
Ali
AuthorHi guys
can you please tell me how to add fade in and fade out effect in trasition.
Guest 00009274
AuthorGreat !
I have a problem trough:
On iOS 6, my original app used to have a navigation controller as it’s initial controller,
Now it’s the empty view controller, and my status bar which used to be translucent (transparent) is now Opaque ! no matter i set it’s style in the app delegate, in info.plist, in target settings or in the storyboard, it’s keeps being opaque.
Any solution ?
Samuel J Ab
AuthorCan someone halp!? Would be lovely to have this enhanced for IOS 7.
dee
AuthorI followed your tutorial , but my side bar view’s background color is not the same as it’s tableview, but I fixed this problem by setting the background color of each table view cell . thanks anyway. 🙂
10mitri
AuthorI would like passing data between the front and the rear view controller but I don’t know how… My sideBar it’s a dynamic menu (json feed) and I would like to charge all item’s menu in the view did load of my front view controller (main page). How can I pass this item?
Thank you for your help!
10mitri
AuthorI tried to use the segue “sw_front” but it doesn’t work…
darko
Authorhello
great job, i found it useful. i have one problem… before reveal view controller i have few more (for example login and the like) and in sidebar view controller i have item “go back”. on clicking it it should jump back to one of previous view controllers (before reveal view controller). how it should be done? i couldn’t find “clean” way to do it
Bmatichuk
AuthorIt uses around 30mb in iOS7 and you never get it back. It would be nice if there was a way to free it.
Chanchal
AuthorIf you compile and run the app, you’ll see an app displaying the “News Frontpage”. No, I don’t see News Frontpage, I see the while empty view controller, completely field to implement other things while I am stuck in initial stage.
Rakari
AuthorYou probably forgot to sign the blank view to the SWRevealController
Alex
AuthorI know this might be kind of late, but I had the same issue. If you created your own files, you (like I did) forgot to make the connection with sidebarButton (declared in mainViewController) to the button on the navigation bar.
Ahmed
AuthorI like this tutorial …. but i want to ask if i want to change the sidebar to right ????
Damien
AuthorHi, thanks for this tutorial. That was really helpful. But I found a small bug, if you are on the MapView, you can’t the slide to go back to the menu does not work. You have to click to the button. Otherwise you can not go back. I think, it’s because of the scrolling property of the mapView. Do you have any idea how to fix this ? Thanks.
[email protected]
AuthorI have recently been using the full source code. I have added a couple of pages and now all of a sudden the pages that require info from a URL are taking long to load. What could I do to make this load faster. Maybbe add an Activity Indicator till the page loads??
Daniel Tovesson
AuthorGreat tutorial! Some questions though. When implementing the sidebar it seems like the “swipe to delete” function in individual cells gets disabled. I can set edit-mode so that all cells get into edit-mode but I can’t swipe to delete single cells. Is there any workaround here? Also, how do I disable the view that I pushed to the right when showing the sidebar? It’s annoying to have it active as I have a tableView there that you can click on.
Kartik Andd
AuthorHey, I have the same problem infact I was just about to ask here.
Looking at the code of the RevealViewController, it sort of picks up any horizontal pan gesture….
Perhaps the best thing to do is, when the press the cell, it slides across and shows the options. I think I might do that…..
try the following search
https://www.cocoacontrols.com/search?utf8=✓&q=tableviewcell
Norman Saadé
AuthorFirst of all great tutorial! Everything is working great but when I tried to reproduce the tutorial I did not manage to add the bar button on the view controller “image view controller” and “map view controller”. Can you explain how you added the bar button although there is no navigation bar any more since the controllers are not linked to the navigation controller?
Norman Saadé
AuthorI solved it myself. Here is what you have to do:
– Select the photo view controller
– In the attributes inspector select “Translucent Navigation Bar” under “Top Bar”
– Add a Navigation Item
– Add the Bar Button
– Add the same code of the slidebarButton from the Main View Controller
frank
Authorhello,
I was wondering how does someone go about creating sections (titles) in the review view controller (menu that slides out) without using the storyboards but programmically!?
Mary Herno
Authorquick question, in this tutorial everything was done thru storyboards, how can you program the menu items, like the titles (multiple titles for section in the menu, like facebook) without using storyboards but programming instead?
Rishad
AuthorHow can I add multiple sidebars for different ViewControllers? I have tried to add 2 side bars and it works fine for me but it is making the storyboard messy :-S. For each Viewcontroller I have to add one SWRevealViewController and one SidebarViewController and add the segues for each menu item. Can I not do it programmatically? Thanks
Mary Herno
Authorhow can you create more then 1 title in the menu programmically
Mary Herno
Authortesting
Mary Herno
Authorif i wanted to disbale the touch of the view that slide out of view, but just wanted the side of the view to act like a slide back can this be done with this library?
witsravi
AuthorHI i am new to app development. I use this tutorial with my custom view. Everything is working fine only i am getting extra title bar. Can anyone suggest me where i am make mistake at the time of view design using storyboard.
witsravi
AuthorHI Simon,
Pls help me Out. I am in the between of my project.
Your tutorial is great and it working fine with normal flow. But i need to use it like Facebook. I want slide view show after user login.
1) create a view for login and another view for swreveal view controller.
2) After that i add library files.
3) create views for front view and back view
But after that when i try to make connection between them. But it does not show seque for connection.
So pls suggest me where i am making mistake. Pls see screenshot
Duffy
AuthorHelp and howdy! I’ve run into a small issue that I think most of you should be able to answer. I’m using iOS 7 with Xcode 5. Simply, I have the menu system working and I have the menu button on my main page. When I click on the menu to go to another page, it loads it but I lose the menu button and am pretty much stuck there. I’ve tried copying the button from the main view controller but it doesn’t show up in my storyboard. I understand I need to put the menu button there and slink it to sidebarbutton but I can never get the button to appear. :< Cheers and thanks to anyone that can lend a helping hand on this.
Maziyar
AuthorI’m using iOS 7 Xcode 5. This is how I do for other viewController. I put a Navigation Item, then Bar Button Item inside Navigation Item, and a Button inside Bar Button. Then I set the Image property inside the Button to the icon that I want. Now it has an appearance but doesn’t do anything. Create an IBAction to your .m file or .h file and call revealToggleAnimated. It will look like this: – (IBAction)myLeftBarButton:(id)sender {
[self.revealViewController revealToggleAnimated:YES];
}
It works fine on all of my viewControllers.
Blake
AuthorI am running core data on my initial view controller and the app delegate is set to display my Table View Controller first which works..Problem is when I try and add these other views before it and change the app delegate to load the other view controller it crashes and gives me an NSInvalidArgumentException’, reason: ‘+entityForName: nil is not a
legal NSManagedObjectContext parameter searching for entity name
‘Player…any idea how to make this work?
Jan
AuthorI have one problem and am in need of assistance. Hi, i tried to add the SWRevealViewController to my project. This is how i did it. First i drag and drop a view controller and i made it the first and initial view of my project and then i attached the SWRevealViewController to it. And following what was done above, i pulled out a table view controller and i linked both the table view and the navigation controller(that was the initial view before implementing the ‘SWReveal’) with a custom segue from the view attached with the SWReveal. And then, just as it was shown above to add some code to the view controller after the navigation controller, i did so exactly.
Before talking about my problem, this is the summary of what was done to my project to implement the sidebar navigation menu, there are only two views that i added to my project and that is the empty view controller and a table view controller, both in which i attached the SWRevealViewController and created a file with the interface as “SideBarController : UITableViewController” respectively. Also i added the two segues to both the table view controller and navigation controller and included the needed code unto my own created view that is positioned as the to-be loaded view after the navigation controller.
Now my problem is that when i load the simulator, i did not go to the view that was positioned in the storyboard to be the view after the navigation controller. Instead i think that i am stuck viewing the view that has the SWRevealViewController attached to it. Is there any way to fix this? Did i forgot to do something? Thanks.
Thabib
Authorits partially working for me. If i select any cell fro slide bar it is navigating to that view but from that view i cant able to come back to main view the left bar button item is not visible at all…. Help me if any one knows the solution
Efrain
AuthorI have used the tutorial and all is working fine. I tried to use a TableViewController to show information once a item on the SideBar menu is clicked. However, it doesn’t show the contents of the table. I did create a ViewController.h/.m for this view similar to the MapViewController.h/.m. Im not sure if there something I should add at SideBarViewController.m prepareForSegue in order for the app to manage segues to a TableViewController. I would appreciate any help or suggestion.
lks.
AuthorHey, great Tutorial 🙂
When I’m doing this like the tutorial I dont have any problems.
But I want to do a GroupeStyled Menu.. And than is the app crashing :/
Here the error:
2014-02-13 22:03:58.109 test2[7475:70b] *** Terminating app due to uncaught exception ‘NSRangeException’, reason: ‘*** -[__NSArrayI objectAtIndex:]: index 1 beyond bounds [0 .. 0]’
*** First throw call stack:
Has anyone an idea how to fix this? :/
Mrithula
AuthorHi, This tuitorial is great.. But I have a problem when I put a navigation controller say Controller A and push the SWRevealViewController. when i swipe from the edge of the FrontviewController it swipes to my controller A. 🙁 Did anyone get such a situation?
kevin
AuthorThe following error appears when i try to launch after the first steps of the tutorial.
Last step: If you compile and run the app, you’ll see an app displaying the “News Frontpage”. But that’s it. You won’t see the sidebar menu when tapping the list button. We haven’t implemented the action method yet.
I can run the app but i get a complete white screen.
Meanwhile I get the following output from Xcode:
2014-02-19 11:54:07.200 SidebarDemo[566:13d03] Unknown class SWRevealViewController in Interface Builder file.
I hope someone can help me
linuxito
AuthorHello, How can implement a second level for this menu?
Yasser Jennani
AuthorGreat tutorial , it works perfectly
Tim Foerster
AuthorHi ! I made all the view controllers I need and made the sw_rear and sw_front segue. In the tutorial it says the app would now show the main view controller (the news page) when I try to run it. But mine doesn’t, it only shows a black screen. Please help me, I’ve tried really hard to figure it out myself but it seems like I did everything like in the Tutorial
RockStar
AuthorMention that sw_rear and sw_front in identifier… works for me… while i had that black screen problem
بو طلال
AuthorI have Same issue , its only show black screen at the same step
Lalo Cura
AuthorI get the same only black screen, I guess the problem is when the segue are created from the blank area of the SWRevealViewController to the Sidebar view controller and to the Navigation controller
danman
AuthorI get the same black screen, I think something’s changed in the latest version of SWRevealViewController and this tutorial is now falling foul of it. Annoying that you spend ten minutes setting everything up before getting here…
danman
AuthorSussed it – specify SWRevealViewControllerSegueSetController not the push version for the segues.
Oliver
AuthorThanks, danman. follow your suggestion, I already fix this issue, but just first step.
Cristian González
AuthorI found the problem: You must change the class of the Navigation Controller to SWRevealViewController, the same as the UIViewController. 🙂
For me it works and in the book (for me) says:
First, select the empty UIViewController and change its class to “SWRevealViewController”.
[…]
Repeat the same procedures to connect SWRevealViewController with the navigation controller.
Hahahaha
tobitech
AuthorI don’t seem to understand your solution. could you please make it simpler. I couldn’t change the Navigation controller class in xcode, it defaults back to UINavigationController
Michael M
AuthorFor those who want to use navigation controllers in their SidebarViewController just change the code in prepareForSegue of the SideViewBarController to this:
– (void) prepareForSegue: (UIStoryboardSegue *) segue sender: (id) sender{
if ( [segue isKindOfClass: [SWRevealViewControllerSegue class]] ) {
SWRevealViewControllerSegue *swSegue = (SWRevealViewControllerSegue*) segue;
swSegue.performBlock = ^(SWRevealViewControllerSegue* rvc_segue, UIViewController* svc, UIViewController* dvc) {
UIViewController *newVC =[segue destinationViewController];
[self.revealViewController setFrontViewController:newVC];
[self.revealViewController setFrontViewPosition:FrontViewPositionLeft animated:YES];
};
}
}
This is a modified version of Jeremy’s answer. Thanks Jeremy.
Mark
AuthorCan anyone help me with this question relating to this tutorial?
http://stackoverflow.com/questions/22581458/adding-bar-button-item-to-left-of-navigation-item
Tander
AuthorAwesome tutorial.
Just one question: In a UiTabBar application, where my front view is my UItabBar and my side bar is a different controller – how can I send data to the side bar menu – when I open the slide out menu? Since prepareForSegue is not called?
Santosh Maharjan
AuthorIn the picture, when I click section B, i want to close slider menu. How can i achieve that? Thank you
Hales ❤
AuthorSAME
HiVoid
AuthorHi, this is a great tutorial, and I really appreciate it. I initially disabled user interaction for some of the static cells. I want to enable them later programmatically. How can I access those static cells? Thank you!
Jerome sahayadarlin
AuthorI keep getting an error in my console saying:
*** -[__NSArrayM insertObject:atIndex:]: object cannot be nil
And this is the line of code that my apps stops running:
[self.view addGestureRecognizer:self.revealViewController.panGestureRecognizer];
achien
AuthorHello! Is it possible to add a blank slide out view on the right side of the main screen in addition to an existing slide-out navigation panel on the left? Thanks!
Elohim
AuthorYes you just have to add another UITableView, connect it as the first
one but assign its segue as sw_right, instead of sw_rear. And in the .m
file of the class tht manage the ViewController where you wanna have 2
menus..in the viewDidLoad method, set the .action of the button as
@selector(rightRevealToggle:); instead of @selector(revealToggle:);
nilkash
AuthorHi I tried this one every thing is going well. But I stuck at last point when I tried to do this inside prepare for segue
[navController setViewControllers: @[dvc] animated: NO ];
it gives me following error:
2014-04-11 12:31:29.382 DemoSlideView[2897:60b] -[FrontView setViewControllers:animated:]: unrecognized selector sent to instance 0x8c498a0
2014-04-11 12:31:29.416 DemoSlideView[2897:60b] *** Terminating app due to uncaught exception ‘NSInvalidArgumentException’, reason: ‘-[FrontView setViewControllers:animated:]: unrecognized selector sent to instance 0x8c498a0’
Thank you for such great tutorials. Thank you.
nilkash
AuthorHi I tried this one and its working fine. Only thing it stuck at last point. when I tried to replace ui view inside prepare segue like this :
[navController setViewControllers: @[svc] animated: NO ];
it gives me following error:
2014-04-11 12:31:29.382 DemoSlideView[2897:60b] -[FrontView setViewControllers:animated:]: unrecognized selector sent to instance 0x8c498a0
2014-04-11 12:31:29.416 DemoSlideView[2897:60b] *** Terminating app due to uncaught exception ‘NSInvalidArgumentException’, reason: ‘-[FrontView setViewControllers:animated:]: unrecognized selector sent to instance 0x8c498a0’
If I remove that one that one it works fine. but it is not replacing ui view. Need help.
Thank you for such great tutorials. Thank you .
DoctorCobweb
Authorgreat tutorial. very helpful. indeeeed.
Ali
AuthorGreat Job, but I would like to mention that “rightRevealToggle” is not working 🙂
cantona
AuthorHi Appcoda I’m big fan, I thank you guy’s helps alot great tutorial
noname
Authorthis is a great code and was working really well.
however it is failing on iOS 7.1.1
does anyone have a workaround?
Braves
AuthorThanks!
Vikas Gupta
AuthorGreat Tutorial. In this example, all the menu items navigate the user to a “ViewController”. How about if one of my menu item navigates to a UITableViewController? I tried it and it doesn’t work for me.
Kyle
AuthorIs there any way to return to the main view controller without the controller updating/refreshing itself? (like something along the lines of dismiss view controller?)
Richard
AuthorHi – the dropbox link for the sidebarempty file is giving an SSL error
Manimaran
AuthorGreat thanks to AppCoda team, all your posts are very clear and easy to understand. thanks for the work…
Lokendra Surya
Authorwhat should be segue identifier for Map and news
Francesco
Authorhi, when you start the app is shown the page “news.” How can I change the page (example: Map) programmatically? without requiring the user to do anything? THANKS
TCBV
AuthorGreat tutorial. I just want to ask what I should do to add subcategories in the slide out menu?
arpit agrawal
AuthorHow did you connect that sidebarbutton to the button in main view in storyboard …i tried it using different button but it was not showing the option for sidebarbutton(at the time when we connect button to view controller by dragging)
Aakiv
AuthorHi , Everything is working fine . But How should I add side Menu to Tab Bar View Controller ?? I am stuck on this since days… Please Help.!!!!!!!!!!!!!!!
Guest
AuthorIs there anyway that you can allow the user add cells in the slide-out menu as an example: User creates a new category to categorize different things. If you know how to do this please let me know I really need to know.
Guest
AuthorIs there a way for the user to add more cells in the sidebar.
Quân Chùa
Authori download both template and complete project but i cant open them in xcode.I am using xcode 5.1.1.Any idea? Please help me.
Nikhil M Das
Authorhow can i know sidebar is toggled?
richa pathak
AuthorHi,
I need to add a new view controller which should have slide out menu button on the navigation controller.This should happen on the click event of button added in main view controller.
As it is happening on the selection of cell in sidebar controller the same should happen on the tap of button added on main view controller.
Can anyone guide as this is bit urgent.
Thanks
Guest
AuthorHi,
i want add the SWRevealViewController in the swift app, it’s possible?
Marco Boldetti
AuthorHi,
i want add the SWRevealViewController in the swift app, it’s possible?
Help me please!
Thanks…
richa pathak
AuthorHi Simon ng ,
can you please guide on my issue which i have posted below.
Murali Mohan
Author[__NSArrayM insertObject:atIndex:]: object cannot be nil error is occurred when segue is given from photoviewcontroller to my own view controller. But some times it occurs and some times i do not get this error .Please solve my problem because i am at end of the project.But unexpectedly it is showing this error. I was shocked
mycode in photoviewcontroller is
– (void) prepareForSegue: (UIStoryboardSegue *) segue sender: (id) sender
{
if ([self.userNameTextFeild.text isEqualToString:@””]) {
UIAlertView *av = [[UIAlertView alloc] initWithTitle:@”” message:@”User name is empty” delegate:nil cancelButtonTitle:@”” otherButtonTitles:@”OK”, nil];
[av show];
return;
}
else if([self.passwordTextFeild.text isEqualToString:@””])
{
UIAlertView *av = [[UIAlertView alloc] initWithTitle:@”” message:@”Password is empty” delegate:nil cancelButtonTitle:@”OK” otherButtonTitles:@””, nil];
[av show];
return;
}
status=[self fetchLoginInfo];
if(status) {
if ( [segue isKindOfClass: [SWRevealViewControllerSegue class]] ) {
SWRevealViewControllerSegue *swSegue = (SWRevealViewControllerSegue*) segue;
swSegue.performBlock = ^(SWRevealViewControllerSegue* rvc_segue, UIViewController* svc, UIViewController* dvc) {
UINavigationController* navController = (UINavigationController*)self.revealViewController.frontViewController;
[navController setViewControllers: @[dvc] animated: NO ];
[self.revealViewController setFrontViewPosition: FrontViewPositionLeft animated: YES];
};
}
}
else{
if ([self.userNameTextFeild.text isEqualToString:@””]||[self.passwordTextFeild.text isEqualToString:@””]) {
}
else{
UIAlertView *av = [[UIAlertView alloc] initWithTitle:@”” message:@”login failed” delegate:nil cancelButtonTitle:@”” otherButtonTitles:@”OK”, nil];
[av show];
}
}
}
Sreeshaj Kp
Authorhow can i show the selected cell permenently until select another viewcontroller
Buk
AuthorFollowed the steps, sidebar is working on simulator but does not show on device (sidebar slides in but the table does not show, background just shows grey). Anyone know why this could be happening?
Stephane
AuthorHi, i have the same issue on xcode 6 with iOS8, the background is grey. I don’t know where is the problem !
John
AuthorHi great tut! But can i do it with Swift! I’ve tried and my UITableView display nothing! Please help me! This is my code:
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
var CellIndentifier:NSString = self.menuItems.objectAtIndex(indexPath.row) as NSString;
var cell:UITableViewCell = tableView.dequeueReusableCellWithIdentifier(CellIndentifier, forIndexPath: indexPath) as UITableViewCell;
if(cell == nil)
{
cell = UITableViewCell(style: UITableViewCellStyle.Subtitle, reuseIdentifier: CellIndentifier);
}
return cell;
}
override func numberOfSectionsInTableView(tableView: UITableView?) -> Int
{
return 1;
}
override func tableView(tableView: UITableView?, numberOfRowsInSection section: Int) -> Int
{
return self.menuItems.count;
}
override func viewDidLoad()
{
super.viewDidLoad()
self.view.backgroundColor = UIColor(white: 0.2, alpha: 1.0);
self.tableView.backgroundColor = UIColor(white: 0.2, alpha: 1.0);
self.tableView.separatorColor = UIColor(white: 0.15, alpha: 0.2);
self.menuItems = [“title”,”home”,”profile”,”settings”,”logout”];
}
Pepper
AuthorHey John, you were able to get your reveal button to open menu w/ Swift? My button is calling revealToggle: method on reveal view controller but no movement of sw_front controller. I have my segues set up properly. I had it working in Obj-C, now creating my first Swift app.
Suresh Shiga
Authori am taking uibuttons in rearview when i am clicking button in rearview that related data will be display in the frontview how using storyboard?
Mantosh kumar
Authorhow i can add UIViewController on place of UINavigationController .
Mantosh Kumar
Authorhow i can add UIViewController on place of UINavigationController
Robert
AuthorI have followed the first part and I am having a problem having the icon show up in the right corner what am I missing? when I tap that side it opens and closes just can not get it to show the image
Brigg Thorp
AuthorGreat tutorial. I added this to my project and for the most part, it works. The only thing that I have seen which I can’t figure out a workaround for is this…My main view is a TableView, and I’d like to add the swipe to delete feature. However, it seems that after I added this control to my project, the swipe no longer works. Any suggestions on what to do to get this to work?
Chris
AuthorAnybody know how to solve the issue that scrollviews appear quite a bit below the Nav bar once you implement this? I guess I could alter the constraints for each one but that is slightly tedious in this case.
premal khetani
Authorcan any one tell me how to resize the frontview while we using it in ipad i need to show sidebar will fully cover the screen how it would be possible? reply ASAP
Vimal
AuthorSlide-out Sidebar Menu- how to set it at left side and right side on navigation bar ?
haristos1991
AuthorHello ….. I have one question … If you want to pass data from frontView to rearView , how you do it ?
James
AuthorThanks so much for this tutorial! Its great. I’d like to use this slide out navigation bar in an app, is that OK?
krish
AuthorMy menu is not getting displayed when I click on bar button. Pangesture is happening but table which I have created is not getting viewed… can anyone help…
hassan
Authorits urgent …. when i select and item from menu then it will not go to next view???? i fellow all steps but its still not working…
kim vasquez
AuthorUIImage *reloadImage = [UIImage imageNamed:@”menu.png”];
UIButton *reloadButton = [UIButton buttonWithType:UIButtonTypeCustom];
[reloadButton setBackgroundImage:reloadImage forState:UIControlStateNormal];
[reloadButton setFrame:CGRectMake(0, 0, 28, 20)];
_sidebarButton = [[UIBarButtonItem alloc] initWithCustomView:reloadButton];
[self.navigationItem setLeftBarButtonItem:_sidebarButton];
how can i implement this one? the gesture is working but the button don’t do anything.
Please help me
Rome1111
AuthorI keep getting this error…”Scene is unreachable due to lack of entry points and does not have an identifier for runtime access via -instantiateViewControllerWithIdentifier”. Anyone know how to solve this?
Alain Vandebosch
Authorlook your segue name and your vc indentifier name
Alain Vandebosch
AuthorI have a navcontoller and several vc should call sidebarview. Iwould like to return to sidebarview with the right vc containing the vc who call. It is possible ?
Tindi_
AuthorThe tutorial is excellent. But how do I display another Controller before the Reveal View Controller? For example a login because it only displays a blank screen
Vino
AuthorGood one. I am trying to implement the last bit.ie. to navigate between the screen. But in my side bar controller i couldn’t implement
if ( [segue isKindOfClass: [SWRevealViewControllerSegue class]] ) {
SWRevealViewControllerSegue *swSegue = (SWRevealViewControllerSegue*) segue;
swSegue.performBlock = ^(SWRevealViewControllerSegue* rvc_segue, UIViewController* svc, UIViewController* dvc) {
UINavigationController* navController = (UINavigationController*)self.revealViewController.frontViewController;
[navController setViewControllers: @[dvc] animated: NO ];
[self.revealViewController setFrontViewPosition: FrontViewPositionLeft animated: YES];
};
It keep saying
Unknown type name ‘SWRevealViewControllerSegue’; did you mean ‘SWRevealViewController’?
What will be the error?
monali
Authorgreat tut….But how to changing the height of one row of UiTableViewCell??
Becki
AuthorReally useful tutorial, although I am stuck on the very last bit. When I copy the prepareForSegue method my code doesn’t recognise the SWRevealViewControllerSegue object. Please help!
Phil
AuthorHello Friends, I got the tutorial working but my problem is that I have a LoginViewController, if the User logins in its been pushed to the Navigation Controller towards a master / detail ViewController Setup. I want the Side menu only appear on the Detail View Controller. I did a lot of stuff connecting in my view controller in my story board but i didn’t get working. The Main issue is this:
– (SWRevealViewController*)revealViewController
{
UIViewController *parent = self;
Class revealClass = [SWRevealViewController class];
while ( nil != (parent = [parent parentViewController]) && ![parent isKindOfClass:revealClass] )
{
}
return (id)parent;
}
It always returns nothing if the revealViewController is not lunchviewcontroller.
BillSnebold
AuthorI got all this to work but I’m not crazy about the way the front view bounces to the right before sliding back over. Does anyone know how to change it so it just slides to the left without that bounce animation?
Muku
AuthorHey,Great Tutorial,But found an issue when we start the app and tap the slide-out menu fro first time, Its is showing a lag.
napolux
AuthorWatch out. In latest SwRevealViewController code the class SWRevealViewControllerSegue is deprecated, so it will no longer work if you try to implement the code in the tutorial from scratch.
Simon Ng
AuthorThanks for your comment. You’re right. And we’ve updated the tutorial to reflect the change. It’s now compatible with the latest version of SWRevealViewController.
Nikos Grigoriadis
Authorhi… the source code from the given Git repository seems to have changed… some functions and data types are not used anymore, specially in the last part of the tutorial where the performBlock function is used. Any hints about that piece of code? cheers
Guest
Authoris anyone getting answers ? tons of questions no answers.
Dilip
AuthorHi,
Thank you for providing this library. While I am implementing this content in the table(cell’s) in sidebar is not rendering and also menu icon is not showing up at any time. Is there anything I am missing. I am using Xcode 5 and IOS SDK 8. Please help me with the useful information.
Thank you,
Dilip.
Simon Ng
AuthorDilip, I believe you’re using Xcode 6. Please check again. The tutorial has just been updated for Xcode 6 and iOS 8.
philly
Authorwere someone able to add the slide menu to a detail view controller?
Cyril Ivar Garcia
AuthorHow can I change the UIBarButton properties to a regular button?
Logan
AuthorThanks to you, I used this. Recently I need to load another page at app load according to background event. But currently always first page is shown first. How can I make second page is up first when appdelegate detect some event or notification received?
Hitesh Borse
Authori used this code for my new project. and very good code. implemented successful for iPhone.
but if my app is universal den i have 2 storyborads one for iPhone and other for iPad.
then if i run app on iPad and in
sideViewController when we select any row then prepareForSegue method select iPhone segue. how select iPad segue??
Hitesh Borse
Authormy id:[email protected]
pawandeep singh
AuthorHey nice tutorial , but there is a PROBLEM that I am facing with this library is that for example I am having button in Photo View Controller of your tutorial and when I am in this view and I open the slide menu and when I click on this button it is clickable and it opens up the other segue it is connected to. So how to disable Photo View Controller when slide menu is opened. Please help..
D-UK10
Authorplease release an xcode 6 version to download as i really need it for my project thank you
Simon Ng
AuthorWe’ve just updated the tutorial for Xcode 6 and iOS 8. Please check again.
Hitesh Borse
Authorthanking you for this tutorial sir. i am using this code in to my app. but now i want to add back button to every view. when user press that back button then user will goto previous view. so please tell how can i implement this back button??
email : [email protected]
Tiago
AuthorHi!
Great tutorial, but I believe it needs an update.
The menu button is fading. Or getting invisible, because when running the app, the function to call the menu is active. But the button is “invisible”.
I’m sweating the emulator. Xcode 6 – iOS 8.1 iPhone 5
Simon Ng
AuthorThe tutorial is now updated for Xcode 6 and iOS 8. Please check again.
denniswellson
Author“SWRevealViewController.h” and
“SWRevealViewController.m” is MIT Soft May i used
“SWRevealViewController” function in my app ,my app maybe in
business?
Umaid
AuthorAbove tutorial is good but I am having little issue in calling sidebar function to my View Controller function a ticket raised at stackoverflow , http://stackoverflow.com/questions/27589670/calling-sidebar-controller-delegate-on-viewcontroller
saintjab
AuthorHi, please how do I can the title menu item to any text programmably. For example instead of APPCODA, I want to change it to any user name programmably. Thanks
Siarhei Brazil
AuthorThx for tutorial, and thx for siting on Ray’s tutorial, I wanted to say that it would be great to reveal how to make custom slide-out nav. panel.
Laila
AuthorGreat tutorial! For someone totally new to iOS, this was very helpful and easy to follow along!
Am I be able to change the content in the side bar based on whichever ViewController I’m on? Let’s say, for example, I’m using a tabbed view controller with two tabs: “boy” and “girl” and would like the side menu to change based on which ever tab I’m currently on. How would I do something like that?
Melissa Lynn
AuthorWow. This is great. I am only half done reading through and already have an app with a working sidebar. Awesome template and coding. You’re a lifesaver! Amazing!!!
Tom
AuthorDidnt work. Cant get my app to show the menu, even after following all the given steps. I click on the menu icon and nothing happens. What’s up?
Leo Wu
Authorit looks very quiet here recently ! Anyways, I have two quick questions after downloaded this demo and tried in my xcode 6 :
1) menu image on the top-left corner doesn’t show up
2) why there is always a black bar on the top and title bar overlaps main view. So top isn’t aligned between sidebar menu and main view
Please see my attachment
LAOMUSIC ARTS
AuthorIn Xcode 611 on Mavericks, I have 2 errors:
– Main.storyboard: warning: Unsupported Configuration: Multiple segues with identifier showPhoto
-Main.storyboard: warning: Unsupported Configuration: Plain Style unsupported in a Navigation Item
Ega Setya Putra
AuthorHey,I’m really new to iOS programming.
I just want to ask can I make that menu items with looping? I have an array and I just want to loop the menu items using my array
Thanks
sagar
Authorproblem with following issue:
Fengson
AuthorGreat tutorial, thanks!
Will Von Ullrich
Authorsoo I have this “slide out menu” inside a tab view controller, and it works nicely (minus a few constraint bugs). However, it has a black bar viewed in the screen, as if an imaginary/black tab bar is on top of the tab bar Im already using. Ill attach a picture of my storyboard and my simulator to help. Not sure if this is a glitch inside the SWcontroller preventing the whole screen to be carried through, or something else. Fairly certain it is the SWcontroller though.
Eduard Bonada Cruells
AuthorVery nice and easy tutorial! I just missed a piece on how to customize the slide-out menu, and I just wrote the post this weekend! Check it here if you want to play around with animations, timings, shadows…: wp.me/p4JlOy-7T
Cameryn Smith
AuthorHey does anyone know where the self.revealViewController is hooked up to. Idk where the property is linked too.
bharned3
AuthorIs there a way to add where when clicking the sw_front view controller the navigation slides back into place.
John
AuthorI am look for a way to have the menu do two things:
1) Modify something in the main controller/view (I have a method, but not sure how to get the reference to the “front controller”)
2) Return to the main controller/view programmatically (basically close the menu, but when I choose in code rather than pushing the button on right hand side or performing a gesture)
I’d like to do this without having to segue into something else.
Does anyone know how to do this?
Rezo
AuthorGreat tutorial. But I have a problem with menu table. Cells in table are filled on the fly from some XML file and there could be different actions (different view controllers to link to) required depend on the type of the menu item/cell. I.e. I can not assign segues in storyboard. As far as I know segues can not be created programmatically. So what is the right way to link this event with SWRevelViewController?
Your response will be greatly appreciated
Marcelo
AuthorHow many navigation controllers are? and WHEN/HOW did you add them?
taholder
AuthorThanks for such a great blog post, really informative and easy to follow, and it’s helped my understanding of ios/swift. Unfortunately, it doesn’t go in to performing a manual segue, this is pretty essential really for a number of reasons a) dynamic menus b) loading different screens at startup. No one seems to know the answer to this (or at least hasn’t posted it) for swift. There is a post here http://stackoverflow.com/questions/24676774/prepareforsegue-in-swrevealviewcontroller-with-swift that I think probably originally worked, but due to an interface change no longer does. Has anyone solved this?
Chris
AuthorCan anyone help me with the following problem? http://stackoverflow.com/questions/29893345/storyboard-setup-with-swrevealviewcontroller
rizwan
Authorif we switch between two navigation controls, it reload the view controller ? is there way just popover from sidemenu
Abi
AuthorWhere should I do some changes if I want to get right sidebar menu instead of the left sidebar?
mostafa
AuthorI have same question. anybody can help ?
Abi
AuthorHi Mostafa, I’ve solved this problem. Do you still have the same question, don’t hesitate to inform me, I’ll gladly tutor you.
mostafa
AuthorHi Abi
Thank you very much.
Yes, Can you help me? I have this problem yet 🙁
Abi
Author1. Use [rightRevealToggle:] method instead of [revealToggle];
2. Set the segue identifier as [sw_right] instead of [sw_rear];
3. Drag the original Bar Button Item from left side to the right.
4. Done. That easy. 🙂
Stanley Chia
AuthorIs it possible to make the menu slide on top of the main page? Also, how do you insert a page before the main page? For instance, Facebook app would have a sign in page before the main page and the sliding menu appears. How to insert this sign in page? I’m trying, but can’t seem to get it right. A slight change and the simulation build fails.
dodets
Authorhow to put toggle button in the bottom of device? also can I toggle the menu from tab bar, (so when I tap certain tab bar then the menu shown)?
lemotz
AuthorHi, i keep getting blank white screen… after the first steps to add segues. I’m on XCode 6. Any suggestion is appreciated.
lemotz
Authornever mind.
Alex Blair
Authorgot this to work just fine, but I’m wondering if having multiple segues with the same “sw_front” identifier is going to be a problem?
PhillipOReilly
AuthorHas anyone else had a problem with SidebarViewController not recognizing selectors commands such as [segue.destinationViewController respondsToSelector:@selector(setTitleForViewController:)]?
The selector exists, but I cannot get the SidebarViewController to recognize it.
Dener Araújo
AuthorHi. How can I keep the navigation bar and push only the content?
Vivek Anand
Authorawesome tut
Lexor
AuthorI did get it to work, but there is one problem. The Sidemenu opens only once, for some reason… did anyone have such problems?
stylerepublic
AuthorThe revealToggle doesn’t seem to be working for me? When I tap my sidebarButton, nothing happens? i’ve followed the tutorial to a T! Any idea what’s going on (I’m using Xcode 7, iOS 9)? Is there a change perhaps I’m missing?
Fouad
Authoris it possible to use this for the iphone and use a master/detail approach for the ipad?
Max
AuthorHi
It worked for me, thanks.
Howether, I need to do another slide-out Sidebar in the same App .
How can I do this, because I can only use once the SWRevealViewController, right ?
Thanks
Andy Ibrahim
Authorhi, tutorial works fine been trying get all sort of errors how do a change Photo View Controller to a Web View controller so it shows a webpage
where do i need to edit or add
IBOutlet UIWebView *webPage;
NSString *webSite = @”http://www.facebook.com/lordie”;
NSURL *url = [NSURL URLWithString:webSite];
NSURLRequest *request = [NSURLRequest requestWithURL:url];
[webPage loadRequest: request];
Gabriel Lidenor
AuthorNice work bro. You helped me a lot 🙂
Chathura Lakmal
AuthorAwesome Tutorial. Thank you
Andrew Dobson
AuthorThanks for great tutorial! Also very helpful to show use of GitHub project in an app.
Minor comment… in the PhotoViewController.m file change the imageNamed call…
self.photoImageView.image = [UIImage imageNamed:[NSString stringWithFormat:@”%@.jpg”, self.photoFilename]];
Only image files of type .PNG are directly referenced. All other image file types require the filename extension.
Abdallah Anwar
AuthorCan anyone please tell me the official Apple terminology for the drawer menu discussed in this article?
Nirav Joshi
AuthorHow to change width of sidemenu which draws on tap/swipe ?
Amit
AuthorHow can i change text color and image on select of tablecell.
David Lee
AuthorHi, i am new to iOS. May i now in PhotoViewController portion how do i know which photo refer to which cell.
Thanks
Bhargav
AuthorIt’s awesome buddy, This BLOG/PAGE is very useful for IOS APP Developers.ThankYou……
ch
Authoris this old ? I did exactly what mentioned, I see black page when run, if i undo everything and make UIViewController Class in stead of SWRevealViewController it shows white UIView, i am using xcode 8.1 and iphone simulator 7.1. what wrong i am doing ?
neeko
Authordownload link is not working.Please help
pavan
Authoritz great
I also want to add tab bar controller at the bottom on top of the slide menu, how can i simply do it.
Raviteja Mathangi
Authorgreat but if i click on the News cell ..can i place the another pop-up button like here is Image plz help me….. https://uploads.disquscdn.com/images/ca8433697b379961352b65d966626c2a7d1a22442e609c25bc7ebbd896429b9f.png
Fawas
Authorhow to add the menu.action for a view controller that not listed in menuview list ?
agis alkarim
Authorgood tutorial … I tried it by making the new project, it’s work, but when I combine it with my old project it does not work, and I’ve followed the same steps
the log that appears is “Unknown class SWRevealViewController in Interface Builder file.”
Help me..
onkar
Authorin my application i have login screen first..on login button action i have load front view controller and left side bar menu(using SWRevealViewController)..but i am unable to logout from left side bar..so how to logout when using SWRevealViewController..