Storyboards Segue Tutorial: Pass Data Between View Controllers
This is the second article of our Storyboards series. In the first tutorial, we introduced the Storyboards, which is a friendly feature in Xcode for designing user interface. If you’ve followed the tutorial from start to end, you should already build a simple recipe app with navigation interface. But we left one thing that was not discussed: data passing between scenes (i.e. view controllers) with segue.
First, let’s take a quick look at what we’ve accomplished. Previously, we learnt to use Storyboards to build a few things:
- Embedded a normal view controller in navigation controller
- Created a table view and populate a list of recipes
- Switched from one view controller to another view controller using Segue
And, this is the final deliverable. When the app is launched, it displays a list of recipes. Tap on any of them will bring you to another view, that supposes to display the details of recipes.

Receipe App with Detail Controller
We didn’t implement the detail view which now shows a static label. So we’ll continue to work on this project and polish the app.
Assigning View Controller Class
In the first tutorial, we simply create a view controller that serves as the detail view of recipe in the Storyboard editor. The view controller is assigned with the UIViewController class by default.

Default View Controller – UIViewController
Let’s revisit our problem. The label in the view should be changed with respect to the selected recipe. Obviously, there must be a variable in the UIViewController for storing the name of recipe.
The fact is the UIViewController class only provides the fundamental view management model. It corresponds to a blank view. There is no variable assigned for storing the recipe name. Thus, instead of using UIViewController directly, we extend from it and create our own class (known as the subclass of UIViewController).
In the Project Navigator, right-click the “RecipeBook” folder and select “New File…”.

Create New File in Xcode Project
Choose “Objective-C Class” under Cocoa Touch as the class template.

Select Objective C Class
Name the class as “RecipeDetailViewController” and it’s a subclass of “UIViewController”. Make sure you uncheck the option of “With XIB for user interface”. As we’re using Storyboards for designing the user interface, we do not need to create a separate interface builder file. Click “Next” and save the file in your project folder.

Create a RecipeDetailViewController class (Subclass of UIViewController)
Next, we have to assign the RecipeDetailViewController class to the view controller. Go back to the Storyboards editor and select the detail view controller. In the identity inspector, change the class to “RecipeDetailViewController”.

Change View Controller Class
Adding Variables to the Custom Class
We’ve just created our custom view controller class by extending from the UIViewController class. However, it doesn’t differ from the parent class until we add our own variables and methods. There are a couple of things we have to change:
- Assign a variable (recipeName) for data passing – when user selects a recipe in the Recipe view, there must be a way to pass the name of recipe to the detail view.
- Assign a variable (recipeLabel) for the text label – presently the label is static. It should be updated as the name of recipe changes.
Okay, let’s add these two variables (recipeLabel and recipeName). Select the “RecipeDetailViewController.h” and adds two properties for the interface:
1 2 3 4 5 6 |
@interface RecipeDetailViewController : UIViewController @property (nonatomic, strong) IBOutlet UILabel *recipeLabel; @property (nonatomic, strong) NSString *recipeName; @end |
Go to the “RecipeDetailViewController.m” and add the synthesis for the variables. Make sure you place the code under “@implementation RecipeDetailViewController”:
1 2 3 4 |
@implementation RecipeDetailViewController @synthesize recipeLabel; @synthesize recipeName; |
Establish a Connection Between Variable and UI Element
Next, we have to link up the “recipeLabel” variable with the visual Label. In the Storyboards editor, press & hold the command key and then click the “Recipe Detail View Controller” icon, drag it to the Label object. Release both buttons and a pop-up shows variables for your selection. Choose the “recipeLabel” variable.

Establish the Connection between UI element and Variable
That’s it. Now you’ve linked up the variable with the Label UI element. Any change in the variable will be reflected visually. But there is still one thing left. We want to have the label to display the recipe name. So in viewDidLoad function, we add the following code that sets the label text the same as the recipe name.
1 2 3 4 5 6 |
- (void)viewDidLoad { [super viewDidLoad]; // Set the Label text with the selected recipe recipeLabel.text = recipeName; } |
Try to compile and run your app. Oops! The detail view is completely blank after selecting any recipe. That’s the expected behavior. We haven’t written any code to pass along the recipe name. Yet, the “recipeName” variable is blank that contributes to the empty text label.
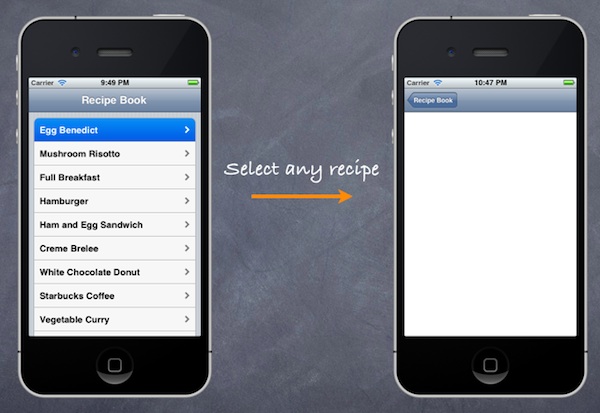
Receipe App with Empty Detail Controller
Passing Data Using Segue
This comes to the core part of tutorial about how to pass data between view controller using Segue. Segues manages the transition between view controllers. On top of this, segue objects are used to prepare for the transition from one view controller to another. When a segue is triggered, before the visual transition occurs, the storyboard runtime invokes prepareForSegue:sender: method of the current view controller (in our example, it’s the RecipeBookViewController). By implementing this method, we can pass any needed data to the view controller that is about to be displayed.
However, the best practice is to give each segue in your Storyboards an unique identifier. This identifier is a string that your application uses to distinguish one segue from another. As your app becomes more complex, it’s likely you’ll have more than one segue in the view controllers.
To assign the identifier, select the segue and set it in the identity inspector. Let’s name the segue as “showRecipeDetail”.

Storyboard Segue Identifier
Next, we’ll implement the prepareForSegue:sender: method in “Recipe Book View Controller”, which is the source view controller of the segue. Select the “RecipeBookViewController.m” and add the following code:
1 2 3 4 5 6 7 |
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender { if ([segue.identifier isEqualToString:@"showRecipeDetail"]) { NSIndexPath *indexPath = [self.tableView indexPathForSelectedRow]; RecipeDetailViewController *destViewController = segue.destinationViewController; destViewController.recipeName = [recipes objectAtIndex:indexPath.row]; } } |
The prepareForSegue method will be called when the transition begins. The 1st line is used to verify the identifier of segue. In this case, the identifier is “showRecipeDetail”. The 2nd line of code invokes the tableView:indexPathForSelectedRow to retrieve the selected table row. Once we have the selected row, we’ll pass it to the RecipeDetailViewController. A Segue object contains the view controller whose contents should be displayed at the end of the segue. You can always use “segue.destinationViewController” to retrieve the destination controller. In this case, the destination controller is the RecipeDetailViewController. The rest of the code is to pass the recipe name to the destination controller.
You can’t run your app right now. After you copy & paste the above method into RecipeBookViewController.m, you should see a number of errors.

prepareForSegue Error
There are three errors as shown above. But we can summarize them into two:
- The property “tableView” is not found in RecipeDetailViewController.
- What’s RecipeDetailViewController? Xcode doesn’t know what it is.
Let’s talk about the second error first. In RecipeBookViewController, it has no idea about RecipeDetailViewController. In Objective C, you use the “#import” directive to import the header file of other class. By importing the header file of “RecipeDetailViewController”, RecipeBookViewController can access the properties and methods of the detail view controller. Place the following code at the very beginning to fix the error:
1 |
#import "RecipeDetailViewController.h" |
Regarding the first error, you should know how to fix it. This is similar to the Label UI element we discussed earlier. There should be a corresponding tableView variable that connects with the UI element.
So, in the RecipeBookViewController.h, add the following code before “@end”:
1 |
@property (nonatomic, strong) IBOutlet UITableView *tableView; |
For the RecipeBookViewController.m, add the synthesis directive to tell the compiler to generate the accessor methods for the tableView variable.
1 2 3 4 5 |
@implementation RecipeBookViewController { NSArray *recipes; } @synthesize tableView; // Add this line of code |
Lastly, go back to the Storyboards and link up the variable with the UI element. In the “Recipe Book View Controller”, hold the command key and click the view controller icon, drag it to the table view. Release both buttons and select “tableView”.
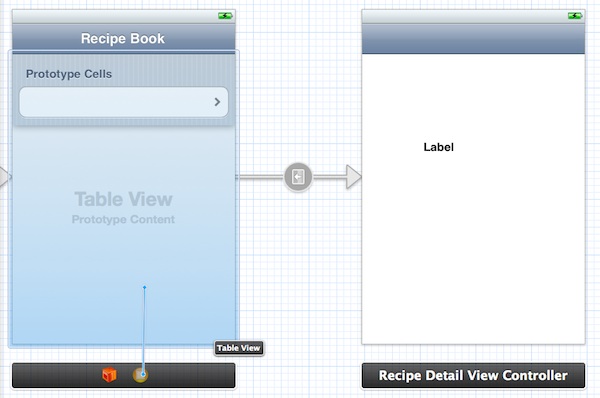
Establish connection with the tableView variable
Now, all the errors should be resolved. Let’s try to compile and run the app. This time, your app should work as expected. Try to select any recipe and the detail view should display the name of the selected item.

Recipe App – Our Final Deliverable
What’s Coming Next?
Isn’t it easy to build an app with navigation interface? With the introduction of Storyboard, it significantly reduces the amount of code you need to deal with. Most importantly, the centralized Storyboard interface provides a high-level overview about the flow of the app. I hope these two tutorials gives you an idea about how Storyboard works and how you can make use of it to build your own app. Though the app we’ve created is simple and with an elementary UI, it elaborates the fundamental concept that you can base on it to build more complex app.
In the later tutorials, we’ll explore the static table cell and continue to make the app even better with tab controller. Stay tuned and make sure you leave us comment below if you have any questions.
Update: You can now download the full source code for your reference.