Chapter 38
Google and Facebook Authentication Using Firebase
Previously, I walked you through how to use Firebase for user authentication with email/password. It is very common nowadays for developers to utilize some federated identity provider credentials such as Google Sign-in and Facebook Login, and let users sign up the app with their own Google/Facebook accounts.
In this chapter, we will see how we can use Firebase Authentication to integrate with Facebook and Google Sign in.
Before diving into the implementation, you probably have a question. Why do we need Firebase Authentication? Why not directly use the Facebook SDK and Google SDK to implement user authentication?
Even if you are going to use Firebase Authentication, it doesn't mean you do not need the Facebook/Google SDK. You still need to install the SDK in your Xcode project. However, with Firebase Authentication, most of the time you interact with the Firebase SDK that you are familiar with. Let me give you an example. This is the code snippet for retrieving the user's display name after login:
if let currentUser = Auth.auth().currentUser {
nameLabel.text = currentUser.displayName
}
You should be very familiar with the code above if you have read the previous chapter. Now let me ask you. How can you retrieve the user's name for Facebook Login? Probably you will have to go through the Facebook SDK documentation to look up the corresponding API.
Let me tell you if you use Firebase Authentication and perform some integrations with Facebook Login (or Google Sign-in), you can use the same Firebase API (as shown above) to retrieve the user's name from his/her Facebook profile. Does this sound good to you?
This is one of the advantages of using Firebase Authentication to pair with other secure authentication services. And, you can manage all users (whether they use email, Facebook or Google to login) in the same Firebase console.
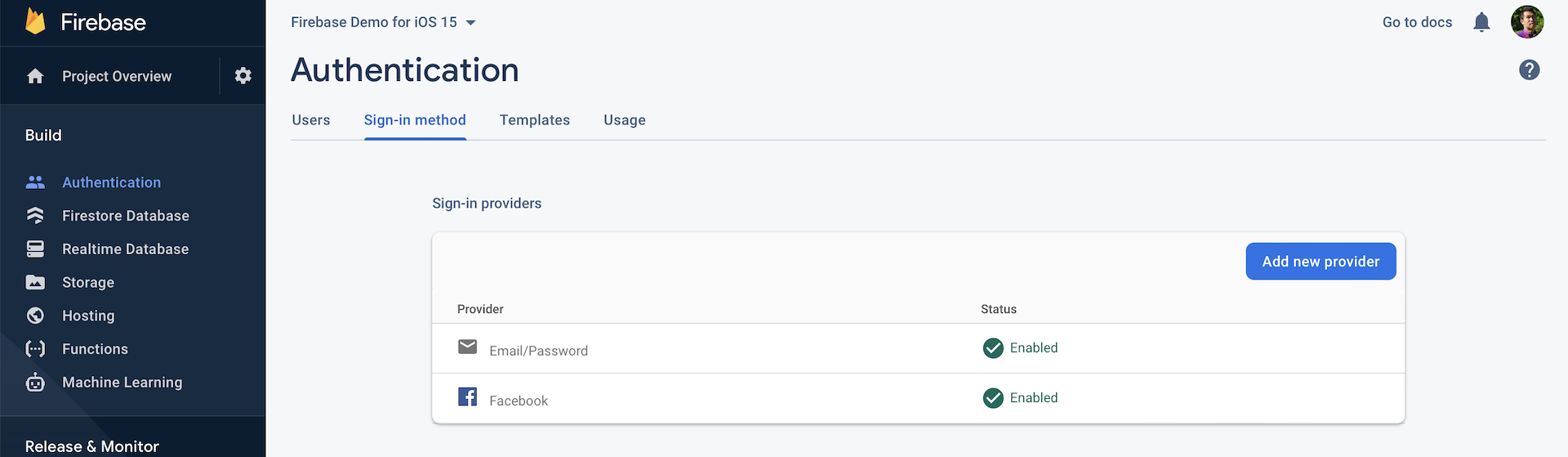
Prerequisite: You better read Chapter 37 before going through this chapter.Now let's begin to talk about the implementation. The implementation can be divided into three parts. Say, for Facebook Login, here is what we need to do:
- Configure your Facebook app - to use Facebook login, you need to create a new app on its developer website, and go through some configurations such as App ID and App Secret.
- Set up Facebook Login in Firebase - as you are going to use Firebase for Facebook Login, you will need to set up your app in Firebase console.
- Integrate Firebase & Facebook SDK into your Xcode project - after all the configurations, the last step is to write code to implement Facebook Login through both Facebook and Firebase SDK.
That looks complicated. I will say the coding part is fairly straightforward, however, it will take you some time to do the configurations and project setup.
Okay, let's get started.
To continue reading and access the full version of the book, please get the full copy here. You will also be able to access the full source code of the project.