Chapter 38
Creating Bar Charts and Line Charts with the Charts Framework
You no longer need to build your own chart library or rely on third-party libraries to create charts. The SwiftUI framework now comes with the Charts APIs. With this Charts framework, available in iOS 16 or later, you can present animated charts with just a few lines of code.
Building a Simple Bar Chart
The Charts framework is very simple to use. In brief, you build SwiftUI Charts by defining what it calls Mark. Here is a quick example:
import SwiftUI
import Charts
struct ContentView: View {
var body: some View {
Chart {
BarMark(
x: .value("Day", "Monday"),
y: .value("Steps", 6019)
)
BarMark(
x: .value("Day", "Tuesday"),
y: .value("Steps", 7200)
)
}
}
}
Whether you want to create a bar chart or a line chart, you start with the Chart
view. In the chart view, we define the bar marks to provide the chart data. The BarMark
view is used for creating a bar chart. Each BarMark
view accepts the x
and y
values. The x
value is used for defining the chart data for the x-axis. In the code above, the label of the x-axis is set to "Day". The y-axis shows the total number of steps.
If you enter the code in Xcode, the preview automatically displays the bar chart with two vertical bars.
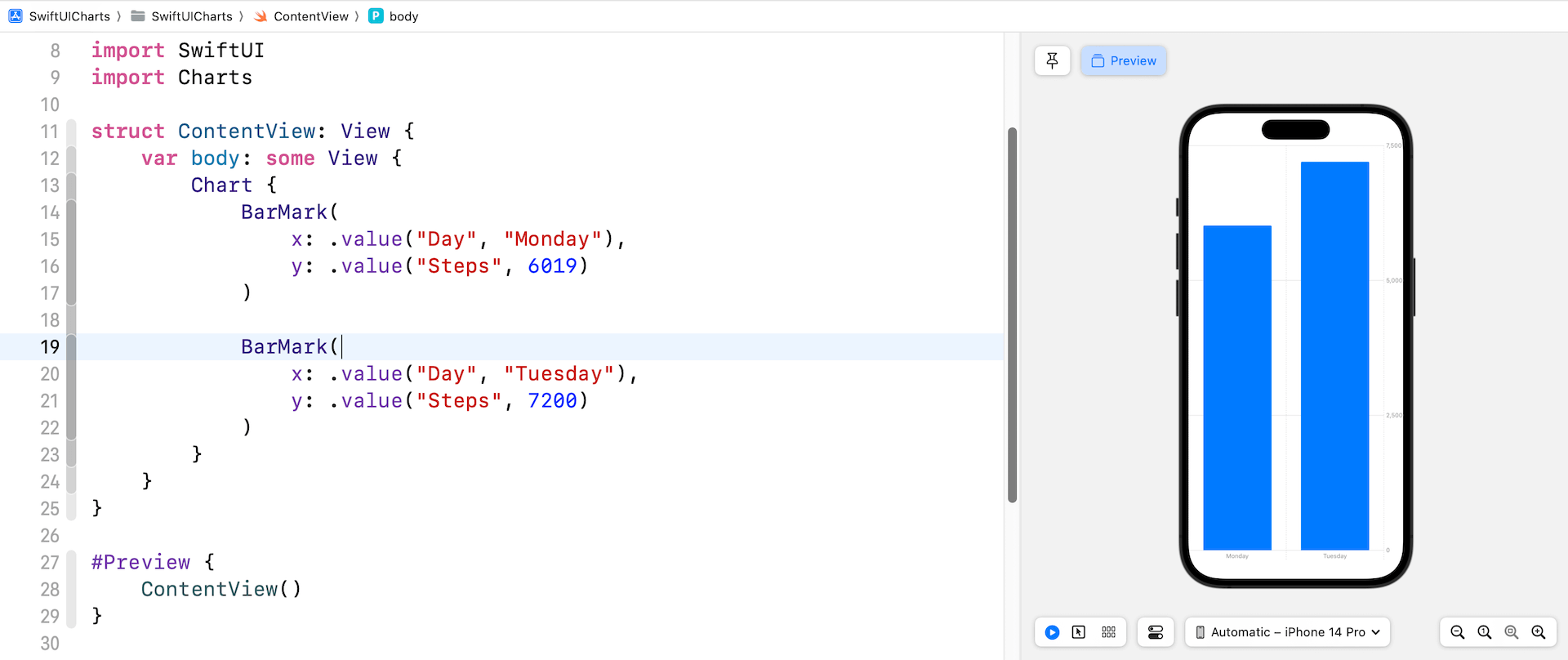
The code above shows you the simplest way to create a bar chart. However, instead of hardcoding the chart data, you usually use the Charts API with a collection of data. Here is an example:
struct ContentView: View {
let weekdays = Calendar.current.shortWeekdaySymbols
let steps = [ 10531, 6019, 7200, 8311, 7403, 6503, 9230 ]
var body: some View {
Chart {
ForEach(weekdays.indices, id: \.self) { index in
BarMark(x: .value("Day", weekdays[index]), y: .value("Steps", steps[index]))
}
}
}
}
We created two arrays (weekdays
and steps
) for the chart data. In the Chart
view, we loop through the weekdays
array and present the chart data. If you have entered the code in your Xcode project, the preview should render the bar chart as shown in Figure 2.
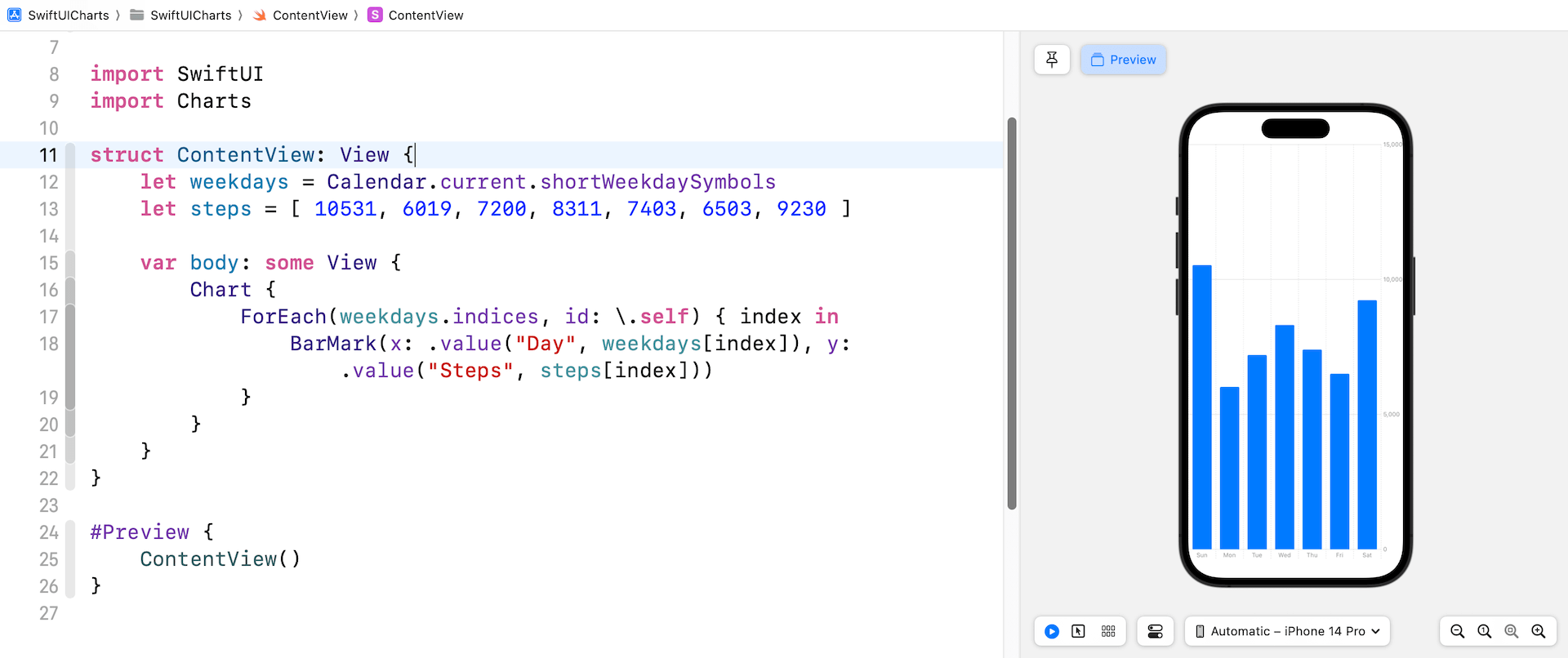
By default, the Charts API renders the bars in the same color. To display a different color for each of the bars, you can attach the foregroundStyle
modifier to the BarMark
view:
.foregroundStyle(by: .value("Day", weekdays[index]))
To add an annotation to each bar, you use the annotation
modifier like this:
.annotation {
Text("\(steps[index])")
}
By making these changes, the bar chart becomes more visually appealing.
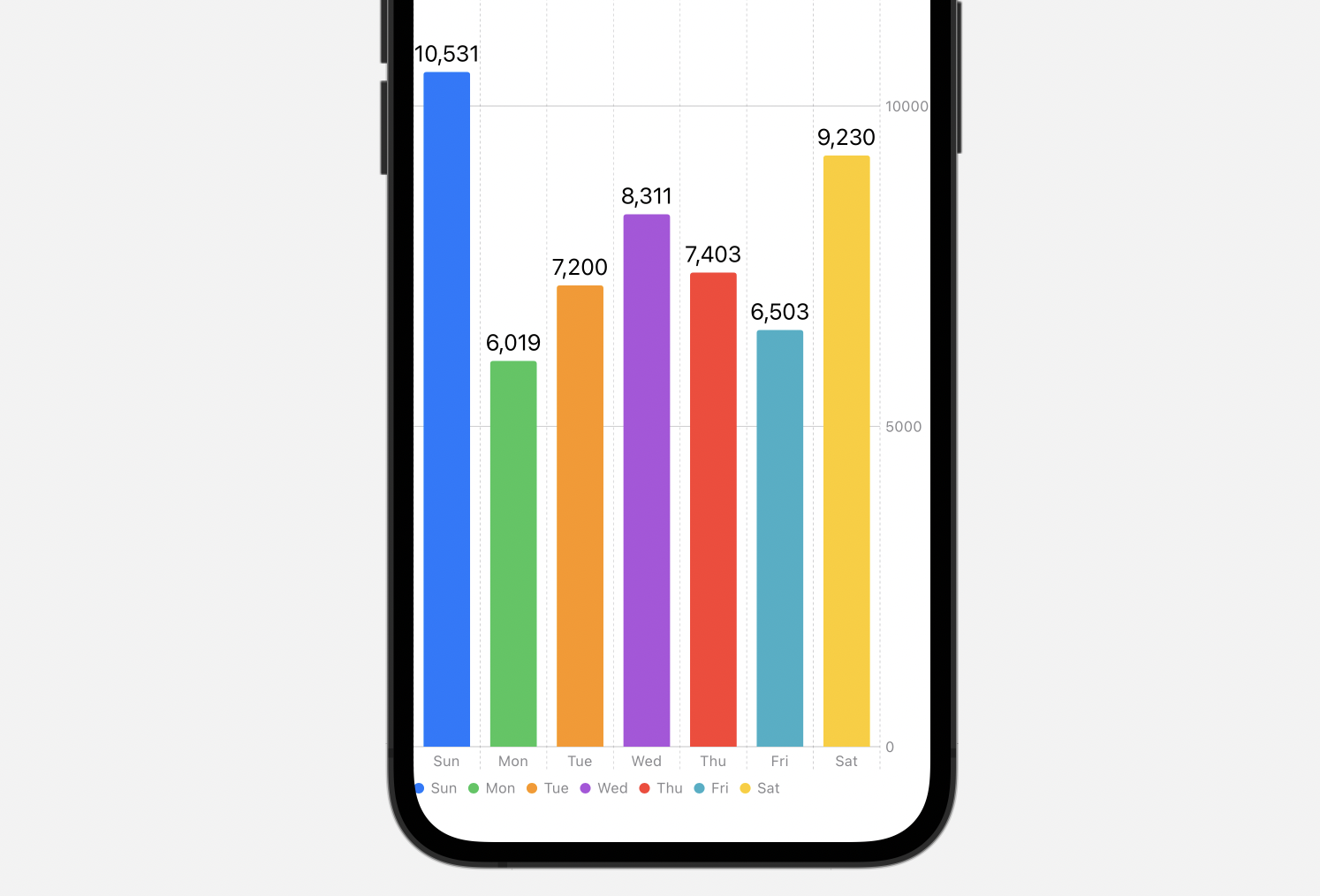
To create a horizontal bar chart, you can simply swap the values of x
and y
parameter of the BarMark
view.
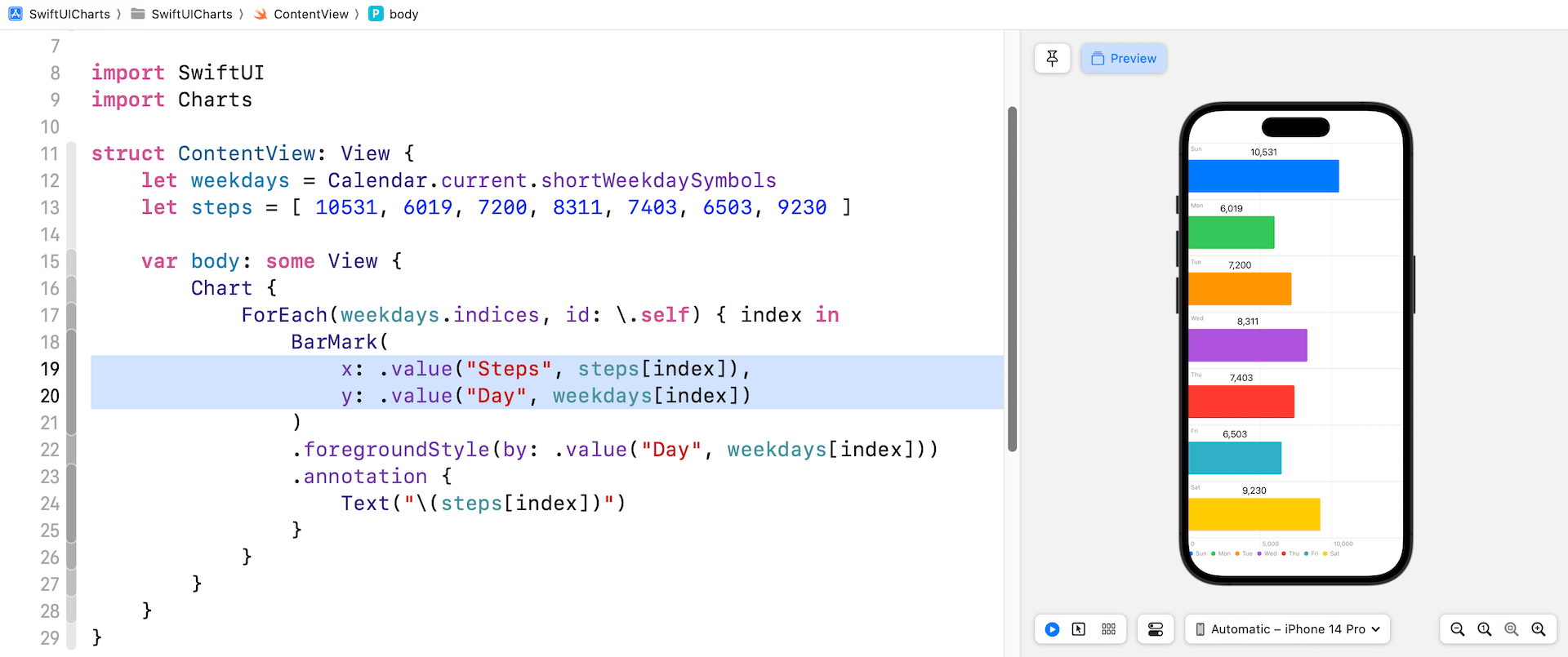
Creating a Line Chart
Now that you understand how to create a bar chart, let's see how to create a line chart using the Chart framework. As a demo, we will create a line chart that displays the average temperature of Hong Kong, Taipei, and London from July 2021 to June 2022.
To access the full content and the complete source code, please get your copy at https://www.appcoda.com/swiftui.