Chapter 53
How to Embed Photo Pickers in iOS Apps
Starting with iOS 16, SwiftUI introduces a native photo picker view known as PhotosPicker
. If your app requires access to users' photo library, the PhotosPicker view seamlessly manages the photo selection process. This built-in view offers remarkable simplicity, allowing developers to present the picker and handle image selection with just a few lines of code.
When presenting the PhotosPicker
view, it showcases the photo album in a separate sheet, rendered atop your app’s interface. In earlier versions of iOS, you couldn’t customize or change the appearance of the photos picker view to align with your app’s layout. However, Apple has introduced enhancements to the PhotosPicker view in iOS 17, enabling developers to seamlessly embed it inline within the app. Additionally, you have the option to modify its size and layout using standard SwiftUI modifiers such as .frame
and .padding
.
In this tutorial, I will show you how to implement an inline photo picker with the improved PhotosPicker
view.
Revisiting PhotosPicker
To use the PhotosPicker
view, you can first declare a state variable to store the photo selection and then instantiate a PhotosPicker
view by passing the binding to the state variable. Here is an example:
import SwiftUI
import PhotosUI
struct ContentView: View {
@State private var selectedItem: PhotosPickerItem?
var body: some View {
PhotosPicker(selection: $selectedItem,
matching: .images) {
Label("Select a photo", systemImage: "photo")
}
}
}
The matching
parameter allows you to specify the asset type to display. Here, we just choose to display images only. In the closure, we create a simple button with the Label
view.
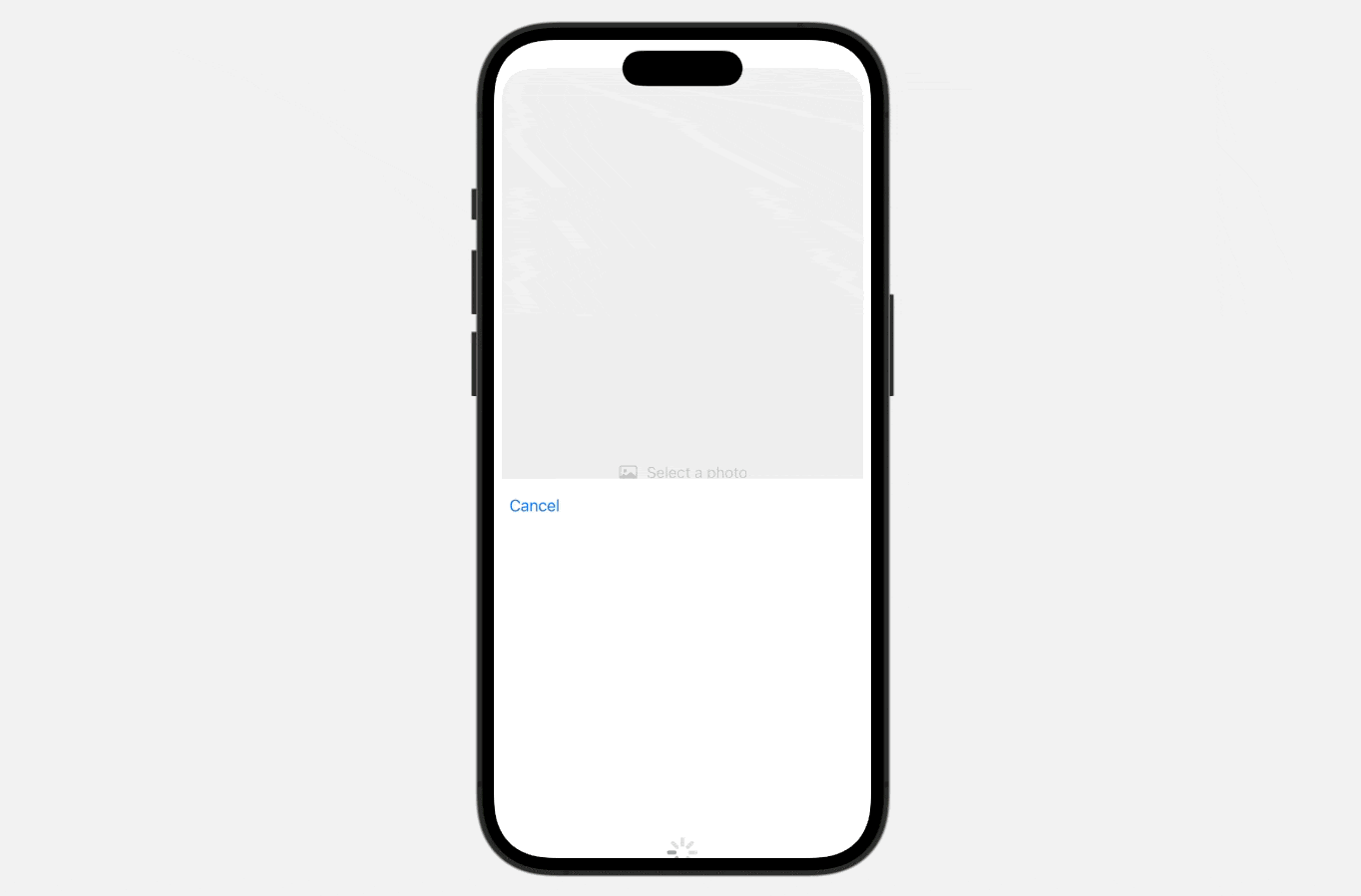
Upon selecting a photo, the photo picker automatically dismisses itself, and the chosen photo item is stored in the selectedItem
variable, which is of type PhotosPickerItem
. To load the image from the item, you can use loadTransferable(type:completionHandler:)
. You can attach the onChange
modifier to listen to the update of the selectedItem
variable. Whenever there is a change, you call the loadTransferable
method to load the asset data like this:
To access the full content and the complete source code, please get your copy at https://www.appcoda.com/swiftui.