Chapter 49
Detecting scroll positions in ScrollView with SwiftUI
One common question that arises when using scroll views in SwiftUI is how to detect the scroll position. Prior to the release of iOS 17, developers had to come up with their own solutions to capture the scroll position. However, the new version of SwiftUI has updated ScrollView
with a new modifier called scrollPosition
. With this new feature, developers can effortlessly identify the item that is being scrolled to.
In this chapter, we will take a closer look at this new modifier and examine how it can assist you in detecting scroll positions in scroll views.
Using the ScrollPosition Modifier
Let’s begin with a simple scroll view that displays a list of 50 items.
struct ColorListView: View {
let bgColors: [Color] = [ .yellow, .blue, .orange, .indigo, .green ]
var body: some View {
ScrollView {
LazyVStack(spacing: 10) {
ForEach(0...50, id: \.self) { index in
bgColors[index % 5]
.frame(height: 100)
.overlay {
Text("\(index)")
.foregroundStyle(.white)
.font(.system(.title, weight: .bold))
}
}
}
}
.contentMargins(.horizontal, 10.0, for: .scrollContent)
}
}
The code is quite straightforward if you are familiar with implementing a scroll view in SwiftUI. We utilize a ForEach
loop to present 50 color items, which are then embedded within a vertical scroll view. If you add this code to a SwiftUI project, you should be able to preview it and see something similar to Figure 1.
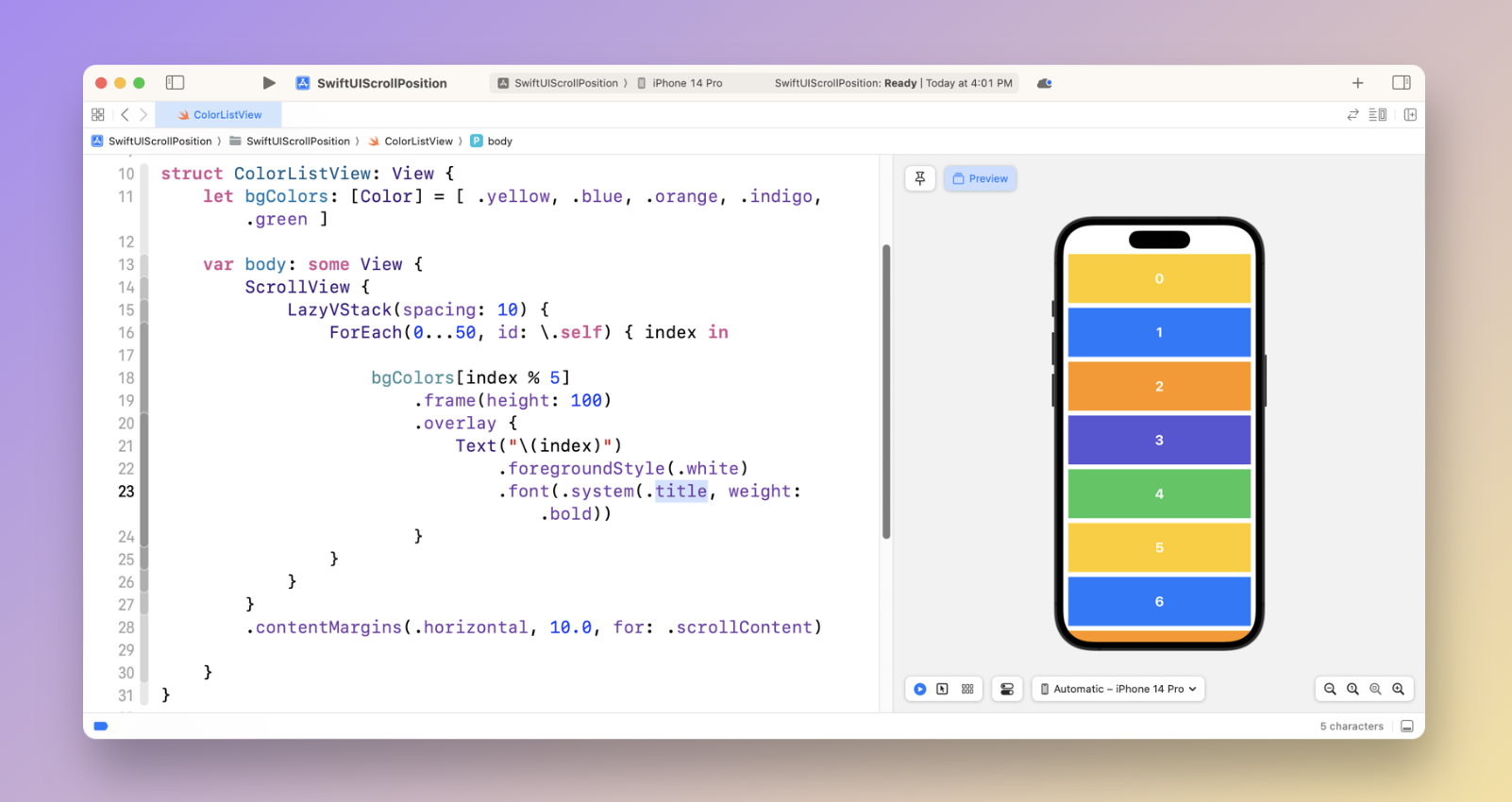
To keep track of the current scroll position or item, you should first declare a state variable to hold the position:
@State private var scrollID: Int?
To access the full content and the complete source code, please get your copy at https://www.appcoda.com/swiftui.